In this post, I’ll show you how to use PHP’s built-in functions to read and print the contents of a CSV file and convert it into an array. We’ll use fopen()
and fgetcsv()
to read the contents of a CSV file, then we’ll convert it into an array using the array_map()
and str_getcsv()
function.
Introduction
Storing data files in comma-separated values (CSV) format is no new thing. In fact, it’s one of the most common ways of storing data due to its simplicity—CSV files are easy to read and write, and can be opened in a basic text editor. This type of file stores tabular data in form of plain text.
An example of such a file would like this:
Elias,40,nurse Rehema,15,programmer Beatrice,23,doctor
This data represents information about three people, with columns corresponding to their names, age, and job. Although it’s a simple data format, it can be tricky to read and consume it.
Therefore, in this article, I will teach you how to open CSV files using PHP’s native functions like fopen()
, how to read the contents of this file with the use of the fgetcsv()
method, and lastly how to convert this CSV file into an array using the array_map()
function.
Of course, there are a number of third-party packages we could use to achieve this, but PHP has built-in native functions that work great.
Prerequisites
To proceed with this article, you’ll need the follwing:
- PHP version 5.6 or above installed on your machine
- a development environment for PHP—either XAMPP or WampServer work well
- some basic understanding of PHP concepts
Let’s begin!
Displaying a CSV File as a Table
In this section of the article, we will be reading a simple CSV file that contains a few words separated by the comma delimiter. For a start, we will use the simple file we used above, then we can later proceed using a random larger file. Of course, you can create your own file using Microsoft Excel or a text editor and save it with the CSV extension.
To read the file, we first have to find it in the folder or location it is saved in. For easier access, you may want to save it in the same folder where you have your program file. Then we can use the fopen()
function to read the file.
Afterward, the fgetcsv()
function checks for CSV fields from a parsed line of the open file. This function requires three parameters: the file handle returned from fopen()
, the maximum length of the line to be read, and the special separator character—which we refer to as the “delimiter”. This can either be a comma or a semi-colon or any other separator.
Now let’s get practical.
Create a file named csvtable.php somewhere it can be served with WAMP or XAMPP and copy the following code.
<?php $file_to_read = fopen('data.csv', 'r'); if($file_to_read !== FALSE){ echo "<table>n"; while(($data = fgetcsv($file_to_read, 100, ',')) !== FALSE){ echo "<tr>"; for($i = 0; $i < count($data); $i++) { echo "<td>".$data[$i]."</td>"; } echo "</tr>n"; } echo "</table>n"; fclose($file_to_read); } ?>
In this file, we’re first opening the data.csv file, which should contain some comma-separated data. fopen
will look for this file in the same folder as csvtable.php. The 'r'
parameter tells fopen
to open the file as read-only.
If the file is opened successfully, fopen
will return a file handle which can be used for other file reading operations. Otherwise it will return FALSE
. Therefore, we check that the file handle is not FALSE
before continuing to read the file.
The fgetcsv()
file then fetches CSV fields from the opened file, one line at a time. We tell fgetcsv
to read at most 100 characters per line, and to use the comma separator as its delimiter. The words found in the file are then looped through and printed into an HTML table.
The last function is the fclose()
function that closes the opened file. This releases the memory used by the open file and allows other processes to have access to the file.
Open csvtable.php through the WAMP or XAMPP localhost server, or run php read.php
from the command line to see the following output:



The first part of what we needed to achieve is done already!
Now, we’ll jump into converting the raw CSV fields into an array.
Converting the Raw CSV File Into an Array
Now, there exists more than one way to produce the array. We can use the fgetcsv()
function to automatically convert the contents of the CSV file into an array, or we can use array_map
.
Using fgetcsv()
to Convert a CSV File Into an Array
This is similar to the example above where we used fgetcsv()
to render a CSV file as an HTML table. Let’s see this in action. Create a PHP file with the following contents:
<?php function csvToArray($csvFile){ $file_to_read = fopen($csvFile, 'r'); while (!feof($file_to_read) ) { $lines[] = fgetcsv($file_to_read, 1000, ','); } fclose($file_to_read); return $lines; } //read the csv file into an array $csvFile = 'data.csv'; $csv = csvToArray($csvFile); //render the array with print_r echo '<pre>'; print_r($csv); echo '</pre>'; ?>
In the above code, we created a function to read a CSV file and convert it to an array. We pass in a parameter with the CSV document file name and path.
Then, we use the feof()
function to check whether the end of file has been reached. Until it has, we need to parse the CSV fields using the fgetcsv()
function. Just like we did in the example above.
The parsed CSV fields from the CSV file are converted into an array using the fgetcsv()
function and are appended one at a time to the $lines[]
variable.
Finally, don’t forget to use fclose()
function to close the opened file before exiting the function.
We then call the readDocument
function that passes the csv document as a parameter and next, the contents of the CSV file are displayed as below:
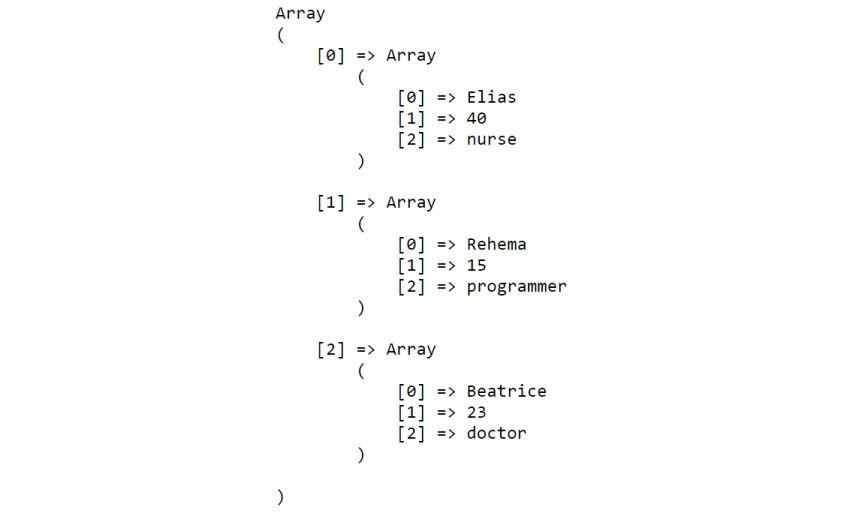
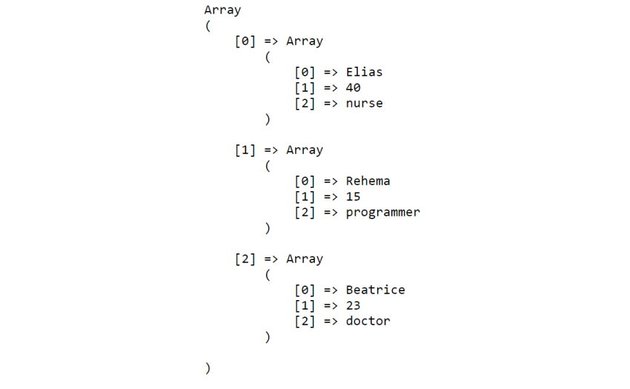
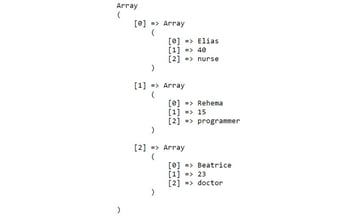
Using array_map()
to Read a CSV File
Alternatively, you can use the array_map()
function to read a CSV file into an array. To do this, you’ll use str_getcsv
as a callback function. This is a built-in PHP function that is used to parse a CSV string into an array.
A callback function is an executable code that is passed to another code as an argument. This argument is expected to be called back later on by the code it is passed to.
Here is how we can use array_map
and str_getcsv
to map our CSV data into an array:
<?php $csv = array_map('str_getcsv', file('data.csv')); echo '<pre>'; print_r($csv); echo '</pre>'; ?>
The above code uses the file()
method to read the csv file into an array of lines. Then, with array map, it calls str_getcsv()
on each line, and store the data for the entire file in $csv
. The str_getcsv()
function that parses the CSV field contents of each line into an array.
The output of the above code snippet will be the same as above.
Notice how much less code is required when using file()
and the the array_map()
function directly?
Conclusion
In this article, you learned how to handle a CSV file in PHP by reading and displaying its contents using PHP native functions like fopen()
and fgetcsv()
and converting the CSV fields into an array. I have showed you two ways to do this.
Now try doing it yourself. Happy coding!