Intents are a fundamental topic for Android developers. It is impossible to build Android applications without coming in contact with intents. In this tutorial, I’ll teach you about intents from A to Z.
What Are Intents?
In a football match, teammates pass the ball around the field with the aim of sending it into the goal of their opponent. The ball is passed from the team’s goalkeeper to their defenders. Next, it finds its way to the midfielders, and if things work out as planned, one of the strikers sends it into the net of the opponent. That’s assuming the goalkeeper of the other side was not able to keep it away!
In Android, the ability to send messages around is made possible by the Intent
object. With the help of intents, Android components can request functionality from other Android components. When you open up the Instagram app on your phone and use it to take a picture, you just made use of an intent. Intents also help communicate between parts of an app; the movement from one screen (activity) to another is made possible by intents.
Look at it this way: all components (applications and screens) of the Android device are isolated. The only way they communicate with each other is through intents.
Starting Activities With Intents
As mentioned earlier, you can use intents to start different components: activities, services, and broadcast receivers.
To start an activity, you will make use of the method startActivity(intent)
.
Here is a code snippet that demonstrates how to start another activity from an intent.
Intent numbersIntent = new Intent(MainActivity.this, NumbersActivity.class); startActivity(numbersIntent);
First, we create a new Intent
object and pass it the NumbersActivity
class. Then we start a new activity using that intent.
Types of Intents
Android supports two types of intents: explicit and implicit. When an application defines its target component in an intent, that it is an explicit intent. When the application does not name a target component, that it is an implicit intent.
Explicit Intent Example
The code snippet of code above is an example of explicit intent. Have a look at it again.
Intent numbersIntent = new Intent(MainActivity.this, NumbersActivity.class); startActivity(numbersIntent);
Here, NumbersActivity
is the target component from our MainActivity
. This means that NumbersActivity
is the defined component that will be called by the Android system. It is important to note (as in the example above), that explicit intents are typically used within an application, because that gives the developer the most control over which class will be launched.
Implicit Intent Example
Here’s an implicit intent:
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.tutsplus.com")); startActivity(intent);
If you have the above code in your codebase, your application can start a browser component for a certain URL via an intent. But how does the Android system identify the components which can react to a certain intent?
A component can be registered via an intent filter for a specific action. Intent filters can be registered for components statically in the AndroidManifest.xml. Here is an example that registers a component as a web viewer:
<activity android:name=".BrowserActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <data android:scheme="http"/> </intent-filter> </activity>
Creating the MainActivity
Layout
The layout for MainActivity
will be very simple for the purpose of this tutorial.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="https://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:orientation="vertical" android:layout_margin="16dp" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Who are you?" android:textSize="22sp" android:textColor="@color/purple_700"/> <EditText android:id="@+id/name_text" android:padding="8dp" android:layout_width="match_parent" android:textSize="22sp" android:layout_height="wrap_content" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Enter your message" android:layout_marginTop="50sp" android:textSize="22sp" android:textColor="@color/purple_700"/> <EditText android:id="@+id/message_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="8dp" android:textSize="22sp"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Your favorite website" android:layout_marginTop="50sp" android:textSize="22sp" android:textColor="@color/purple_700"/> <EditText android:id="@+id/website_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:inputType="textUri" android:padding="8dp" android:textSize="22sp"/> <Button android:id="@+id/submit_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="30sp" android:layout_gravity="center_horizontal" android:layout_marginTop="50sp" android:textColor="@color/white" app:backgroundTint="@color/teal_700" android:text="Submit"/> </LinearLayout>
Here you have three TextView
and three EditText
widgets that prompt you to enter your name, your message and your favorite website. There is also a single button that will take users to a different activity which shows all their data.
The EditText
widget where users will enter the website URL has its inputType
attribute set to textUri
. We have also added unique id
attributes to different EditText
widgets and the Button
to make them accessible in our code.
Using Explicit Intent in an App
Let’s write some code to see how it works out. In this section, you’ll write the code that takes the information provided by user in the MainActivity
and pass it on to ShowActivity
using an explicit intent.
Open up Android Studio and generate your MainActivity
. You can set the name of the package to com.tutsplus.intentdemo.
Your MainActivity
will start with some imports and the class declaration:
package com.tutsplus.intentdemo; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.widget.Button; import android.widget.EditText; import android.content.Intent; public class MainActivity extends AppCompatActivity { }
Then you’ll override the onCreate()
method to initialize the activity with any saved state and the activity layout (we’ll create this later).
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // button handlers go here }
Next you’ll get references to each of the buttons defined in the layout and attach a click listener to each of them.
final Button submitButton = findViewById(R.id.submit_button); submitButton.setOnClickListener(v -> { EditText nameText = findViewById(R.id.name_text); EditText messageText = findViewById(R.id.message_text); EditText websiteText = findViewById(R.id.website_text); String nameString = nameText.getText().toString(); String messageString = messageText.getText().toString(); String websiteString = websiteText.getText().toString(); if(!websiteString.contains("http://") && !websiteString.contains("https://")) { websiteString = "https://" + websiteString; } Intent submitIntent = new Intent(this, ShowActivity.class); submitIntent.putExtra("nameString", nameString); submitIntent.putExtra("messageString", messageString); submitIntent.putExtra("websiteString", websiteString); startActivity(submitIntent); });
For the Submit button, you set an OnClickListener
to trigger an action whenever the button is clicked. When a click occurs, we grab the name, message and website URL, and send them to the next activity: ShowActivity
. We also include a check to see if the provided URL begins with http:// or https:// and prefix it with https:// if that’s not the case.
Finally, we create our intent to display the entered data in another activity called ShowActivity
. The target component is explicitly defined in the intent, making this an example of explicit intent. We use the putExtra()
method to pass along additional information with our intent.
Creating the ShowActivity
Layout
Now lets create a layout for ShowActivity
to display all the information from MainActivity
and two buttons for users to take action.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_margin="16dp" android:orientation="vertical" tools:context=".ShowActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Your Name" android:textSize="22sp" android:textColor="@color/black"/> <TextView android:id="@+id/show_name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="22sp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Your Message" android:textSize="22sp" android:layout_marginTop="30sp" android:textColor="@color/black"/> <TextView android:id="@+id/show_message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="22sp" /> <Button android:id="@+id/send_message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:text="Send Message" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Your Website" android:textSize="22sp" android:layout_marginTop="50sp" android:textColor="@color/black"/> <TextView android:id="@+id/show_website" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="22sp" /> <Button android:id="@+id/open_browser" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:text="Open in Browser"/> </LinearLayout>
This activity contains 6 different TextView
elements. We will use three of them to display information to users. There are also to different Button
elements. The first with id
set to send_message
will take the the message entered by user and help them send it to someone else using apps installed on the device. The second button with id set to open_browser
will open the link provided in MainActivity
in a browser.
Using Implicit Intent in an App
To complete our app, we need to write the code to handle some implicit intents. First, we will get our data from the explicit intent in the earlier section. The code will look like this:
package com.tutsplus.intentdemo; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import android.widget.Button; import android.widget.TextView; public class ShowActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_show); Bundle extras = getIntent().getExtras(); String inputName = extras.getString("nameString"); String inputMessage = extras.getString("messageString"); String inputWebsite = extras.getString("websiteString"); TextView showName = findViewById(R.id.show_name); TextView showMessage = findViewById(R.id.show_message); TextView showWebsite = findViewById(R.id.show_website); showName.setText(inputName); showMessage.setText(inputMessage); showWebsite.setText(inputWebsite); } }
We use the getIntent()
method to get the Intent that started ShowActivity
. Then we use getExtras()
to get access to all the extended data for the Intent. This returns a Bundle
object to us. After that, we use the getString()
method to get the value mapped to our different keys.
You should note that nameString
, messageString
and websiteString
are the keys we used in MainActivity
to store and pass our data. We use the string values returned by getString()
to set the text value of our TextView
elemens.
We will implement the OnClickListener
events for both our buttons now. When create explicit events, we were required to specify the component that we would like to start in order to receive the intent. This is not the case with implicit intents. The system is allows to decide which app or component will receive the intent based on a variety of factors.
A click on the Open in Browser button in ShowActivity
will create an implicit Intent that will open the provided URL in user’s default browser. Here is our code for the OnClickListener
on browserButton
.
final Button browserButton = findViewById(R.id.open_browser); browserButton.setOnClickListener(v -> { Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(inputWebsite)); startActivity(browserIntent); });
We we create out Intent, we pass the action we want to take as the first parameter and the data we want to use as the second parameter. The action in this case is simply ACTION_VIEW
and the data is the website that the user wants to visit.
Now lets implement an OnClickListener
for our Send Message button. It will have the following code:
final Button messageButton = findViewById(R.id.send_message); messageButton.setOnClickListener(v -> { Intent intent = new Intent(Intent.ACTION_SEND); intent.putExtra(Intent.EXTRA_TEXT, inputMessage); intent.setType("text/plain"); Intent chooser = Intent.createChooser(intent, "Send Message Using"); startActivity(chooser); });
This time we set the action to ACTION_SEND
because we want to send the data to someone else. We are not required to specify the person to which we will be sending the data. The createChooser()
method then creates an ACTION_CHOOSER
intent for us. We can optionally provide users some hint on what they are supposed to do now. The users will then select an app from the options shown and send the message to desired recipient.
Testing the App
Now you can build your app and try it out on your Android device! The following image shows what it looks like on my device:
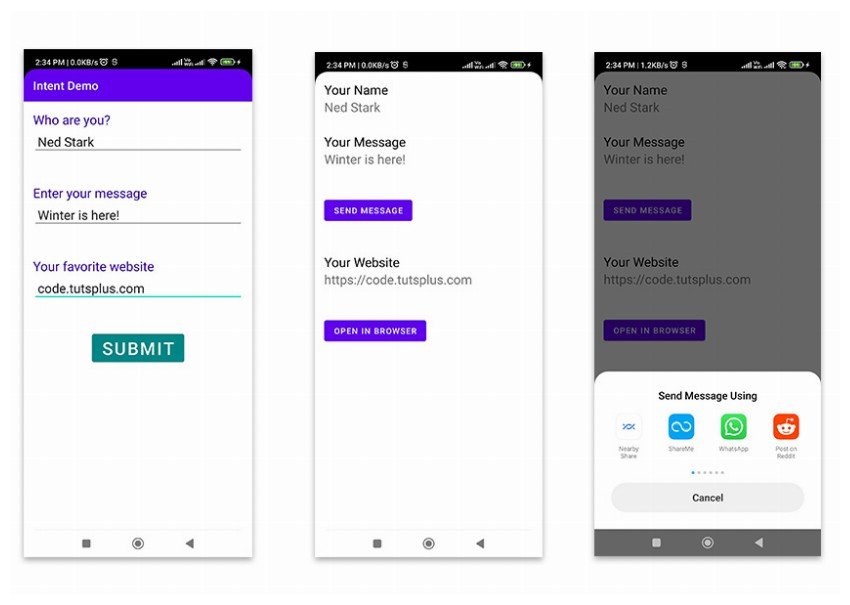
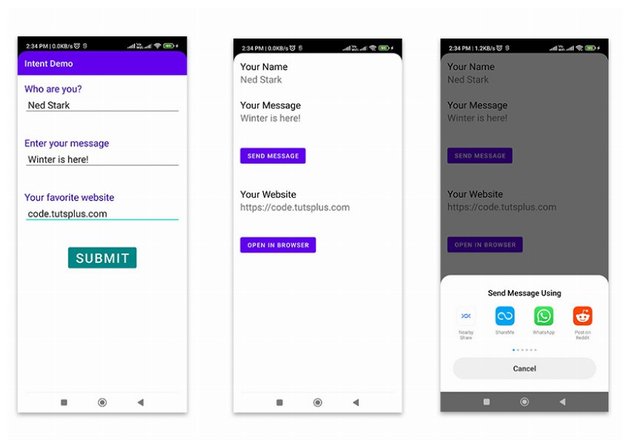
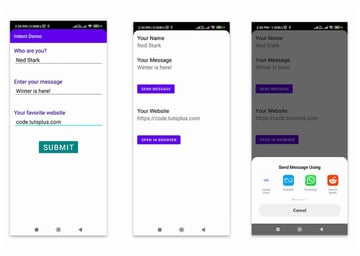
Passing Intent Data Using Bundles
You can also make use of Bundles when passing data via intents.
The Bundle
class allows you store complex data and supports data types such as strings, chars, boolean, integer and so on. Here is an example of how part of MainActivity.java would look if you used Bundle
.
// Creating Bundle object Bundle b = new Bundle(); // Storing data into bundle b.putString("nameString", nameString); b.putString("messageString", messageString); b.putString("websiteString", websiteString); // Creating Intent object Intent submitIntent = new Intent(this, ShowActivity.class); // Storing bundle object into intent submitIntent.putExtras(b); startActivity(submitIntent);
Note that I am using the method putExtras()
here instead of putExtra()
that we used when passing individual data points.
Conclusion
In this tutorial, we got a brief introduction to using intents to create activities in Android. We looked at the difference between explicit and implicit intents, and coded a simple example that used each type.
You can read more about intents in the Android documentation. Understanding how they work is very important. As you build more apps, you will encounter lots of different kinds of Intents and ways of using them.
And in the meantime, check out some of our other posts on Android app development!
Post thumbnail generated by OpenAI DALL-E.