Are you a new developer learning Java? Read ahead to learn how to make your first Java Android app as a beginner.
Creating a mobile application is a big step towards turning your idea into reality. But the primary concern among new developers is how to make an Android app with all the resources at their disposal. If you’re a beginner, you won’t know the purpose of many of them.
So, it is important to begin from the basics and know the starting point.
This guide will walk you through the basics of building an Android app and answer your questions about how to make an app with Java.
We’ll be creating a Java Android app using Android Studio as our IDE (Integrated Development Environment).
How to Make an App in Java
To start making a Java Android app, you have to start with an IDE. There are several options for IDEs, but two of the most efficient ones are Eclipse and Android Studio.
For this guide, we are using Android Studio 3.1.3.
Android Studio is a complete IDE that offers tools that make app development very simple and easy. It has an advanced code editor and several templates for app design. There are tools for development, debugging, and testing as well.
You can learn how to install Android Studio in our post on how to get started making Android apps.
Run Android Studio
Let’s begin with a new project on Android Studio. It gives the option to Create New Project on the welcome screen.
You can also continue with your current project if you have one.
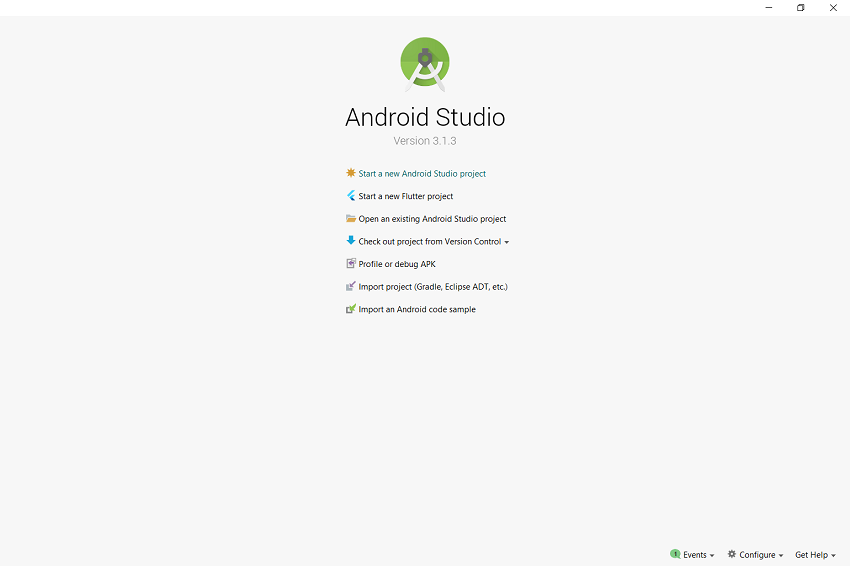
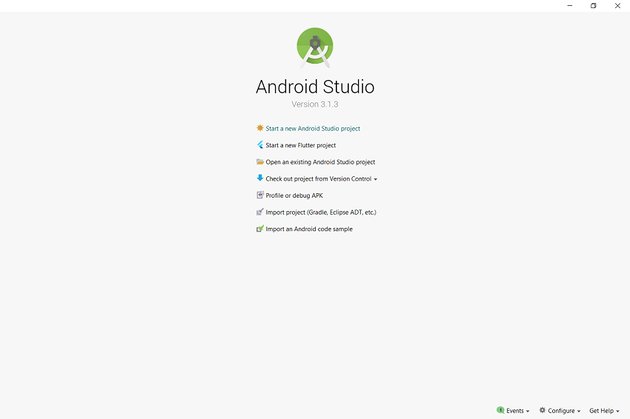
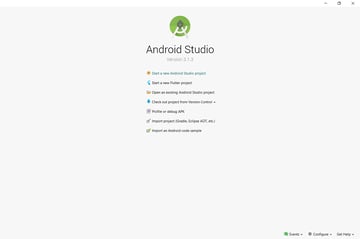
Once you choose to create a new project, the next step is choosing your activity. You will be provided with several options but we’ll begin with Empty Activity for this tutorial on creating a Java app.
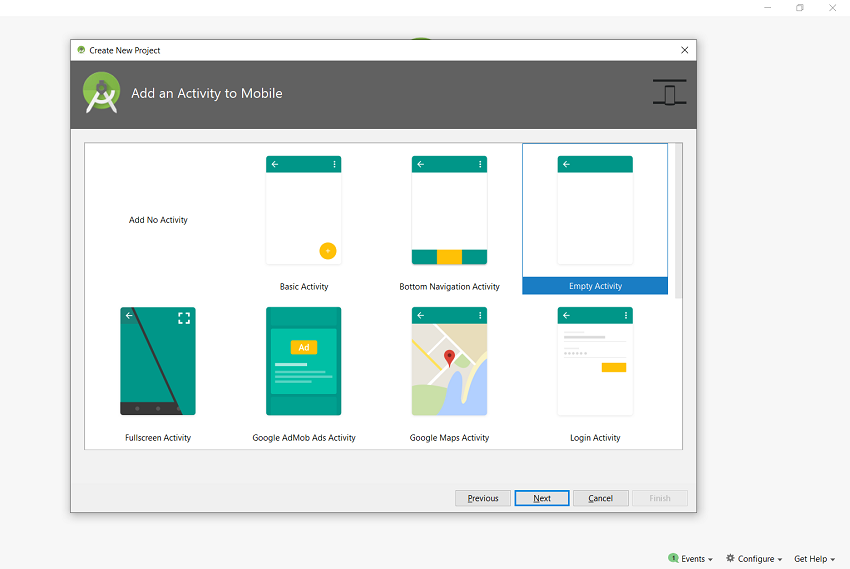
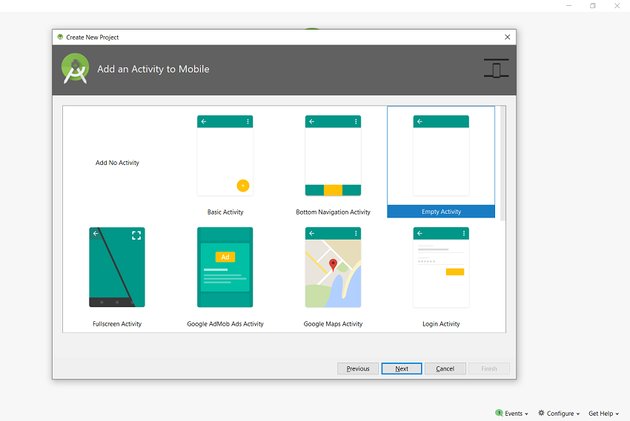
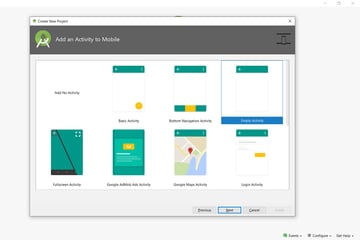
The next step is configuring your activity by giving it a name. Let’s name our app MyBasicApp. From the drop-down menu, choose the Java language. Click Finish.
Now we are ready to start creating our app!
Start Working on Java App Development
At this stage, Android Studio has created two folders that are visible in the left corner. They are:
- A folder for MyBasicApp–this folder carries the code for your app.
- A folder of Gradle scripts–Gradle is a free and open-source tool used by Android Studio to turn the code into an .apk file for your app.
Since we selected the Basic Activity template, Android Studio has created some preset files for our project. You can expand the folders to view them.
Clicking on the app folder will give a drop-down menu with three to four subfolders: manifests, Java, Java (generated), and res. Expanding each one of them will open more folders. Each folder stores a separate component of your project.
In the MyBasicApp folder, go to the Java folder and click on com.example.mybasicapp. This folder contains the source code of your Java Android app.
Now, click the res folder and open the layout folder. It contains a file activity_main.xml. It is the layout of your app. Click to open it.
Now your project view has opened the source code and the layout in two separate tabs. It looks like this:
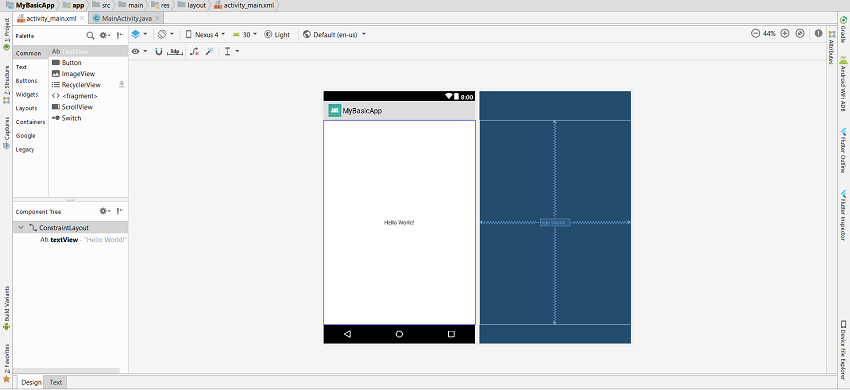
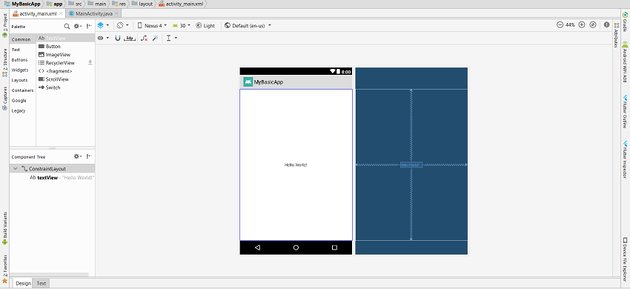
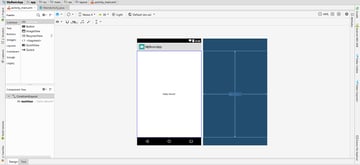
The .xml file gives you a layout where you can drag and drop elements to build your file. You can also change it to code editor by clicking on text at the bottom left corner. Now you can edit the source code instead of adding elements to the design layout.
In the layout editor, the left pane contains all the elements that you can add to the layout. If you see the component tree, there is just one element—Hello World—in our app.
The elements we add to our layout will be shown in the component tree and how they are added in relation to each other.
Here, ConstraintLayout
is the root of the view hierarchy (it is the dimensions of the page on which components will be added).
The ConstraintLayout
has a TextView
called Hello World.
The TextView
is the component that came with a blank activity. The layout at this stage looks like this:
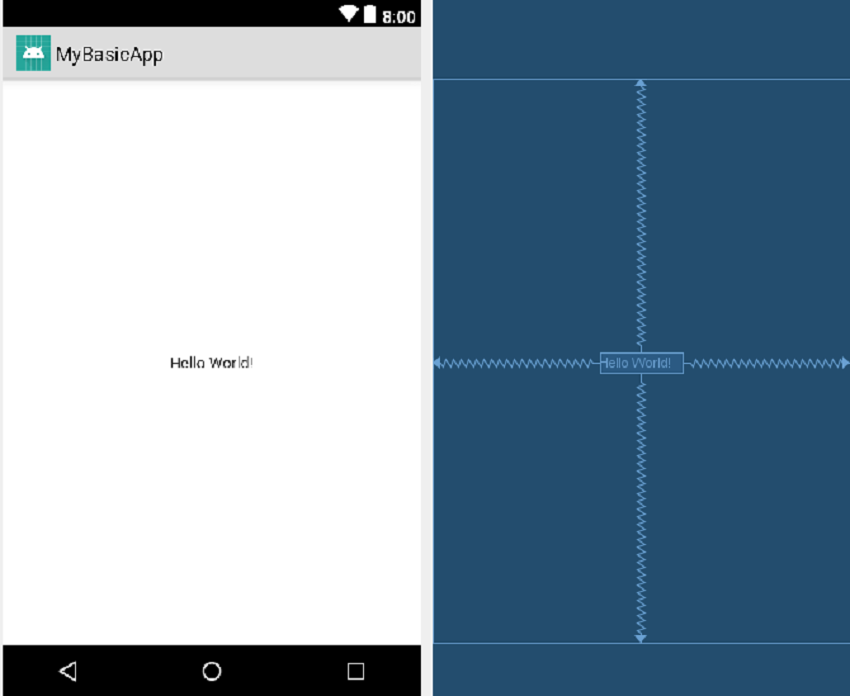
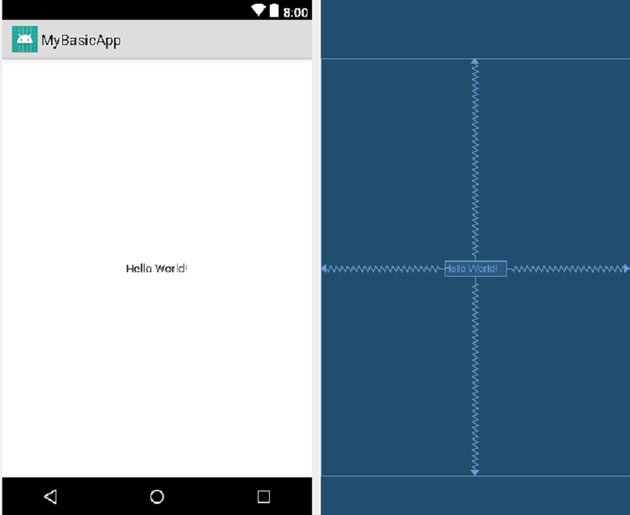
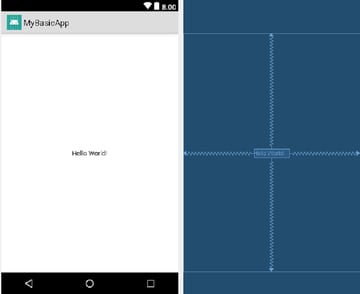
The XML code for this layout will be this:
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> </android.support.constraint.ConstraintLayout>
It has one root hierarchy with just one text element.
Note that this is the basic layout that Android Studio generated itself. We haven’t made any modifications to it yet.
Modify the App Layout
The next step is to modify the layout by adding components of your choice and associating activities with them.
Here you can either start with this ConstraintLayout
with TextView
in it, or you can clear the ConstraintLayout
and begin with a clean slate in terms of layout.
Let’s say you want to change the text on the TextView
. You can go to the code editor and view the properties of the TextView
element. The code will be like this:
android:text="Hello World!"
Change the string to whatever you like. For now, I’ll stick with Hello World.
If you have set up an Android Virtual Device (AVD) in the Studio, the app will run on the simulator like this:
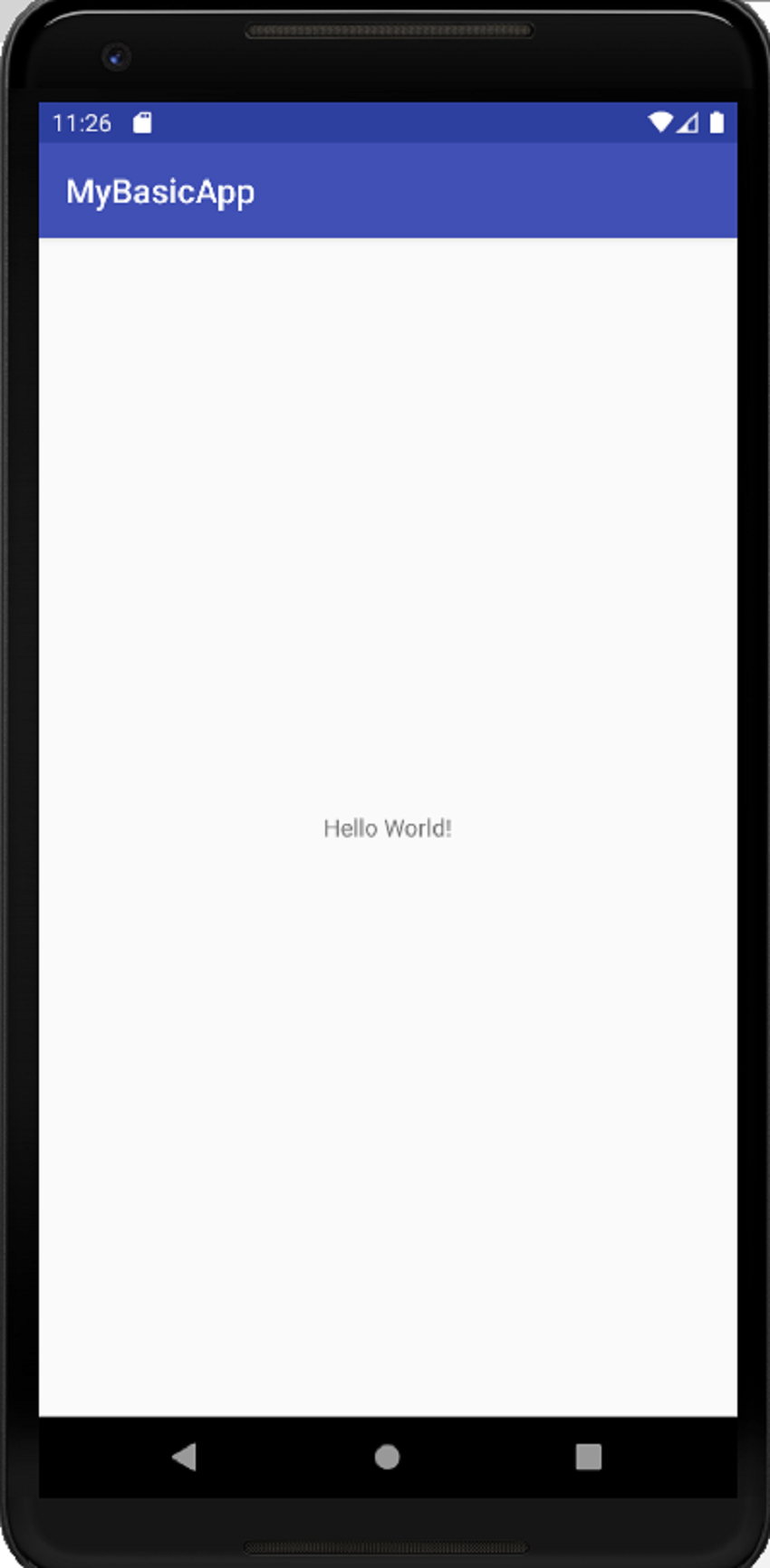
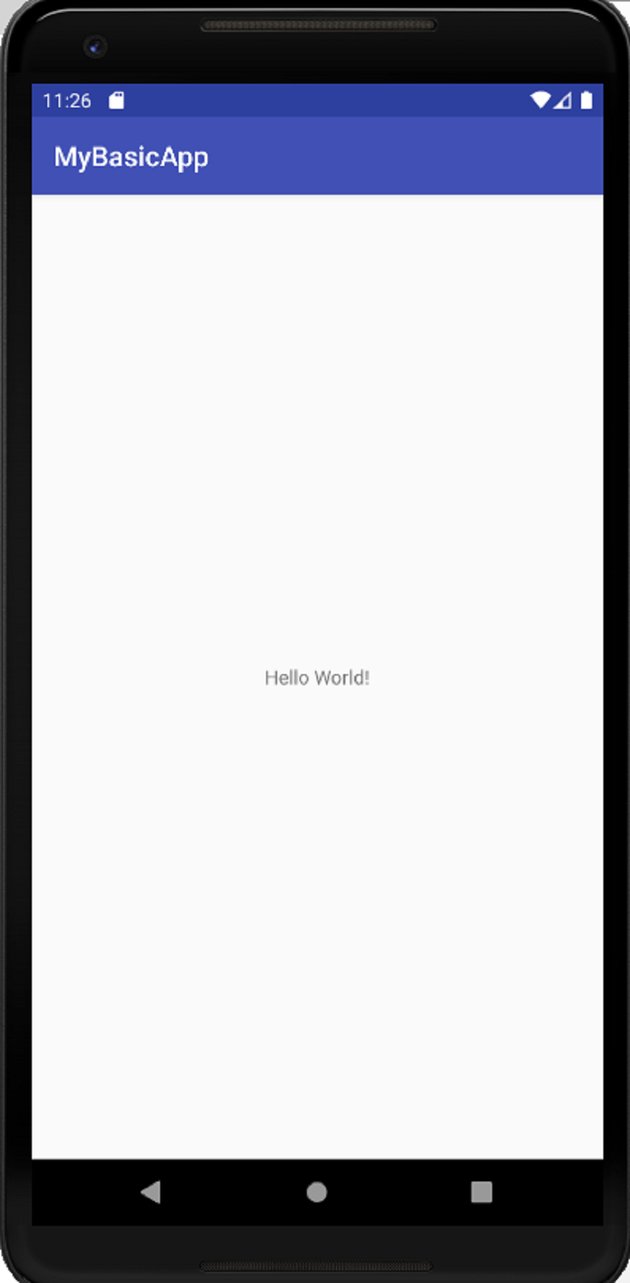
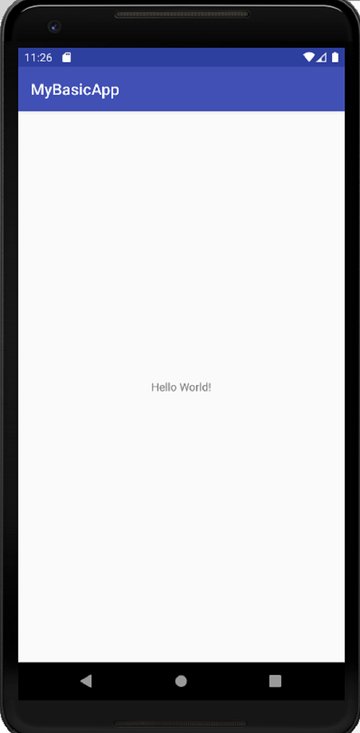
This is the basic TextView
with Hello World!
as its string. You can modify the attributes of this text component. Edit the text, font, size, and color to give a new look to TextView
.
The XML code for these changes would be this:
<TextView android:id="@+id/textview_first" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@color/colorPrimaryDark" android:fontFamily="sans-serif-condensed" android:text="Hello World" android:textColor="@android:color/white" android:textSize="30sp" android:textStyle="bold" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" />
We have changed the font to Sans Serif and made the color darker gray
. The font size is also increased to 30sp
, and the text is made bold.
The changes as visible in the AVD:
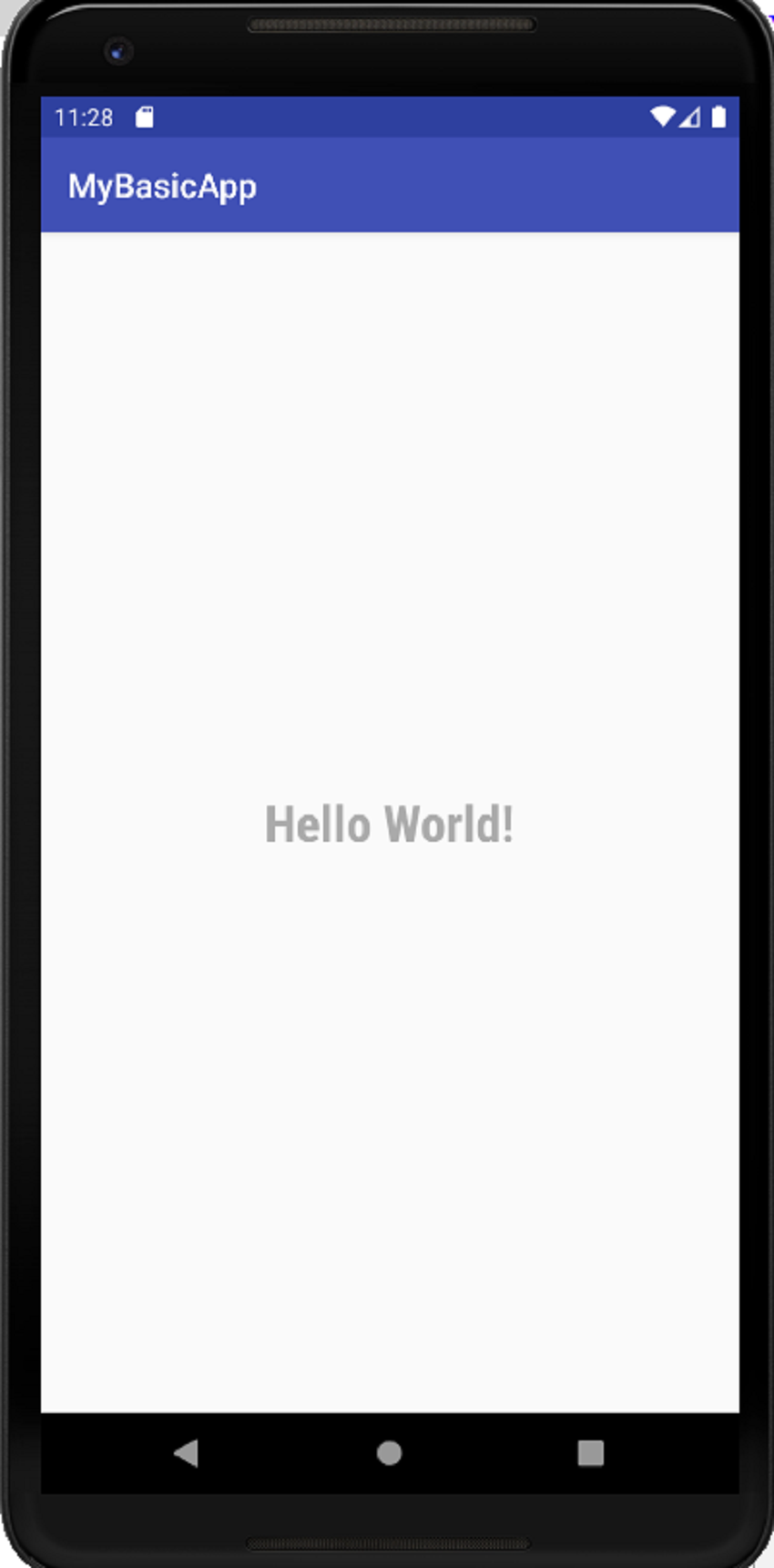
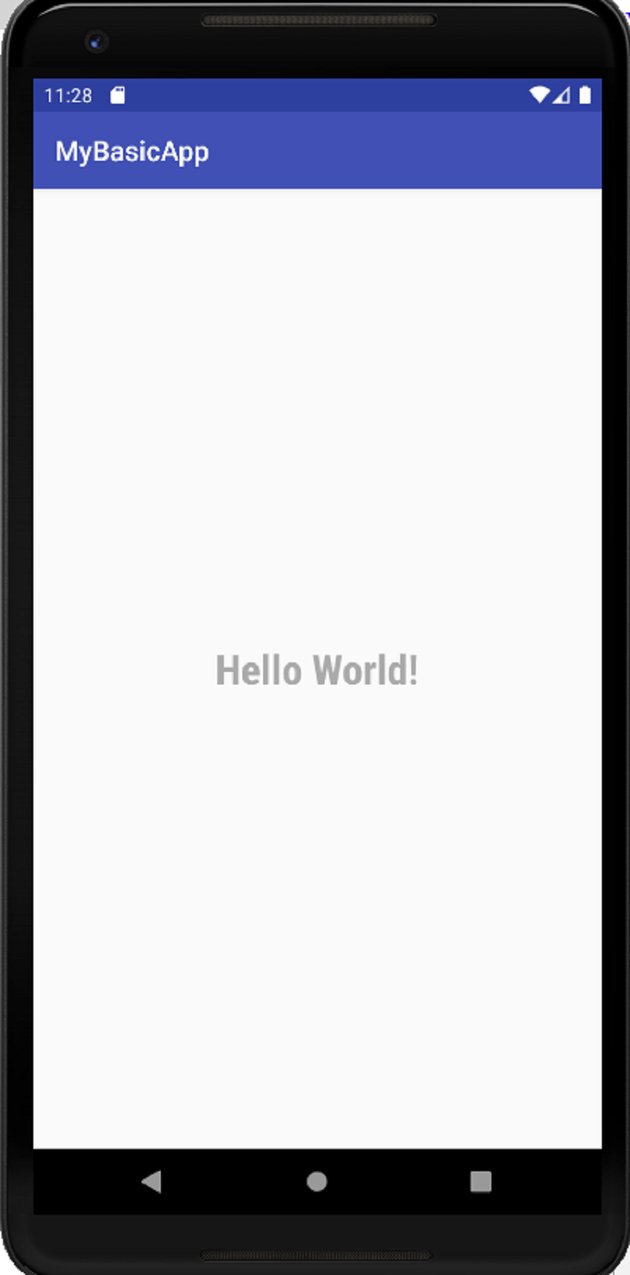
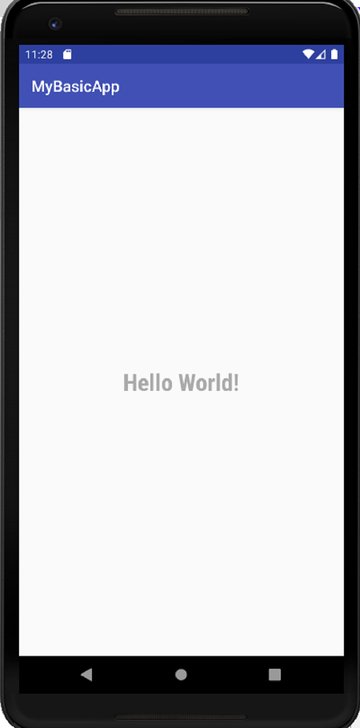
Add Constraints
Up to now now, we’ve just modified the stock Empty Activity set up by Android Studio. Now, we will see how to add constraints and views to the user interface.
In the Layout Editor, there is a palette on the right side from where you can choose the constraints you’d like to add.
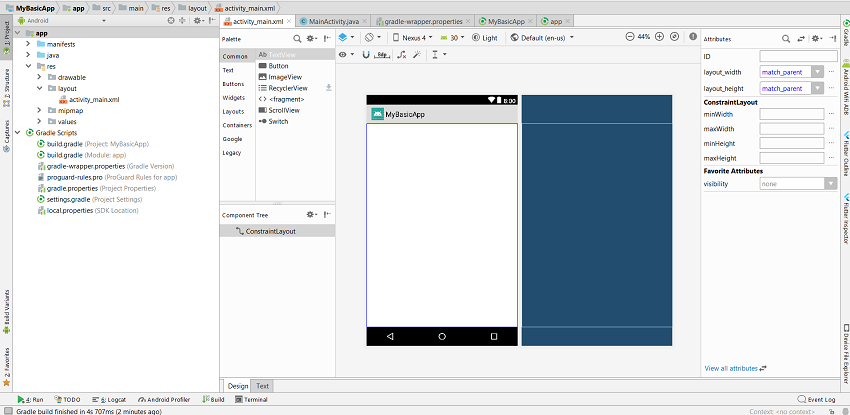
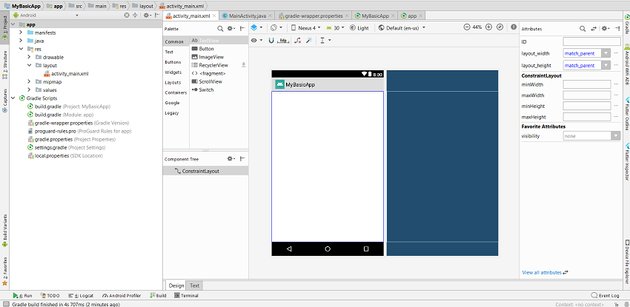
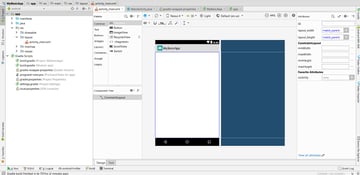
You can add more than one view and constrain them to top, bottom, left, or right. These views have attributes that can be edited to modify them.
To better understand what an attribute is, select textView
in the Component Tree and look at the Constraint Widget in the Attributes panel.
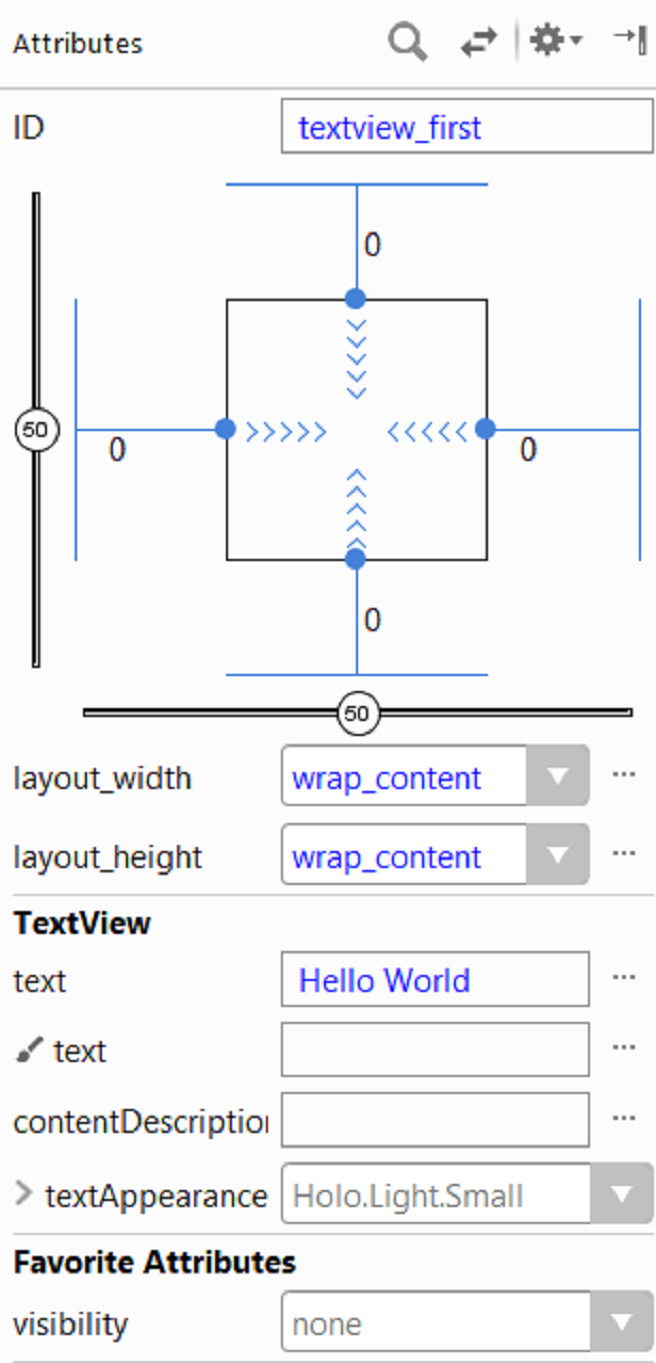
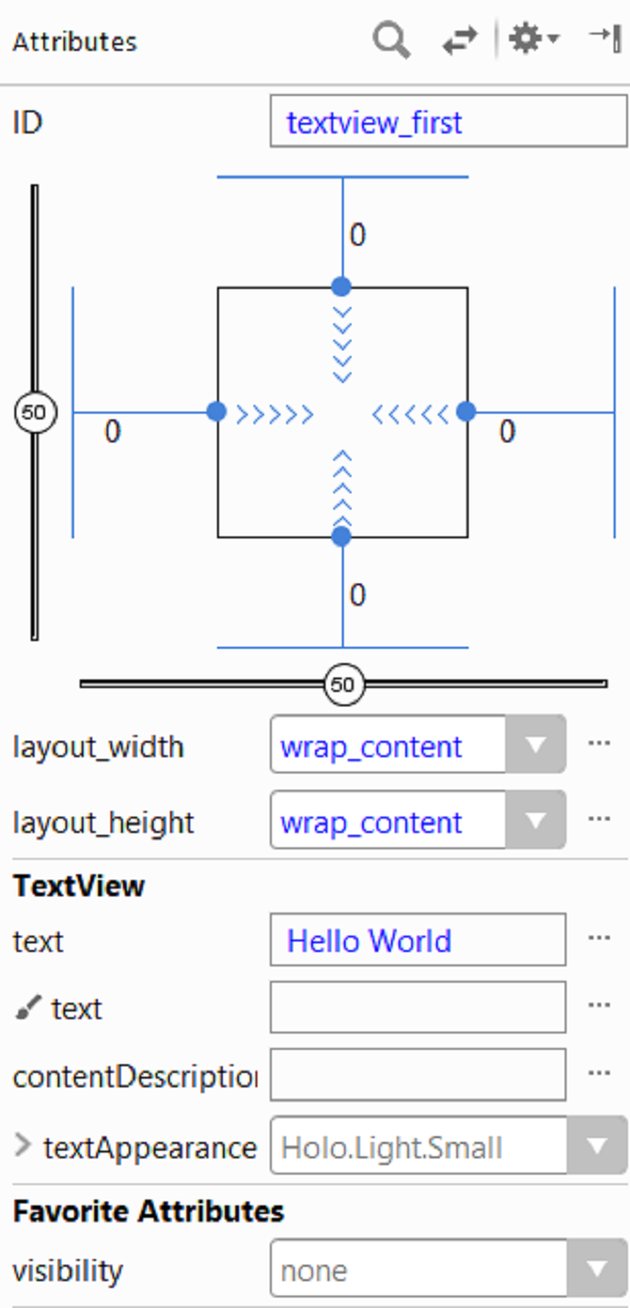
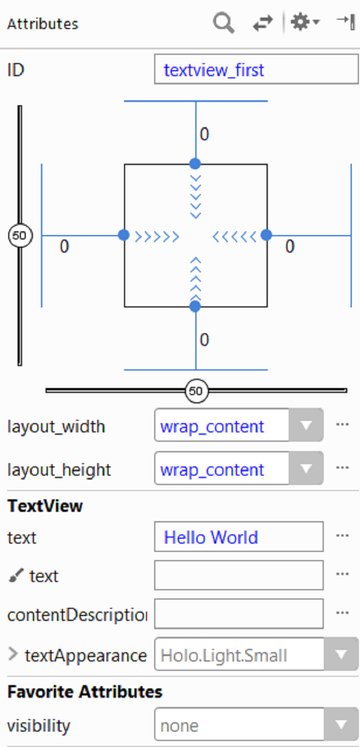
The square represents the constraints.
The rectangular box and each of the four dots represent a constraint. You can increase or decrease their value based on the point where you want to place the view.
Here is how it looks in the Layout Editor.
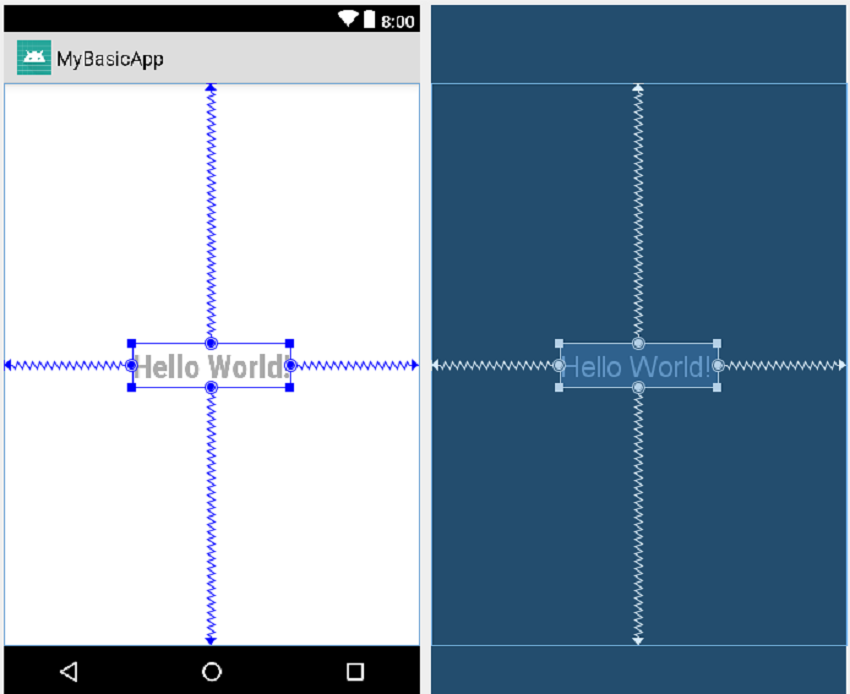
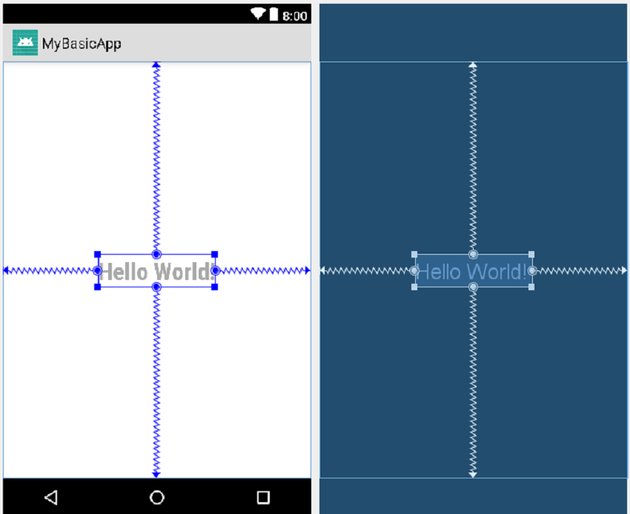
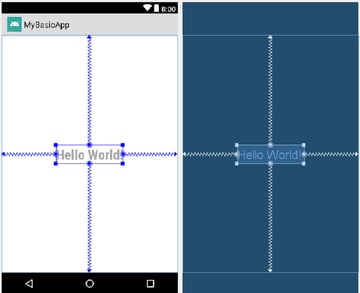
You can drag and drop the Views from the left palette to add them in the ConstraintLayout
. After adding them, you can apply constraints to determine their position. Each new button added to the layout is given a unique identity.
Here is how your layout will look after adding the constraints to the views:
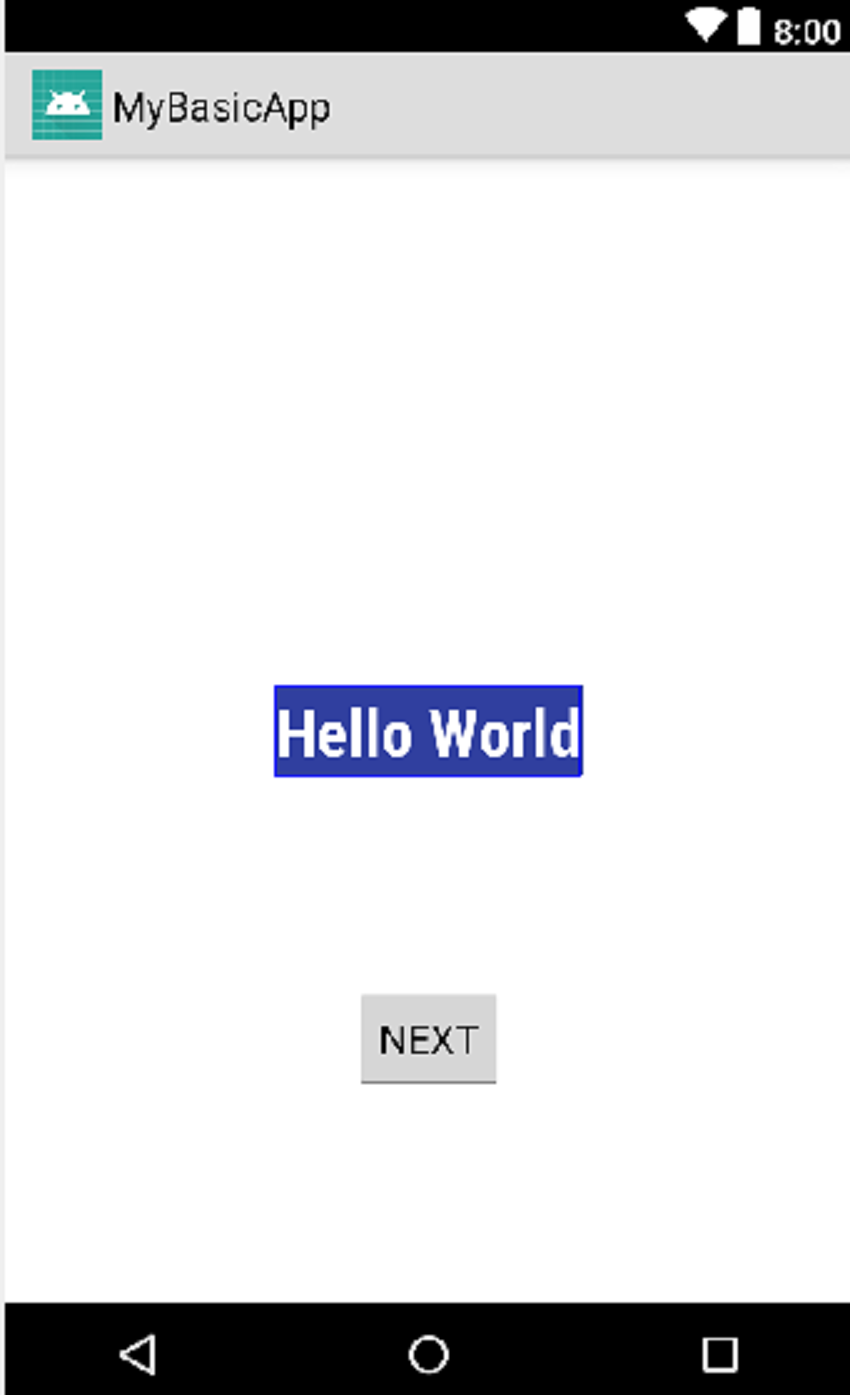
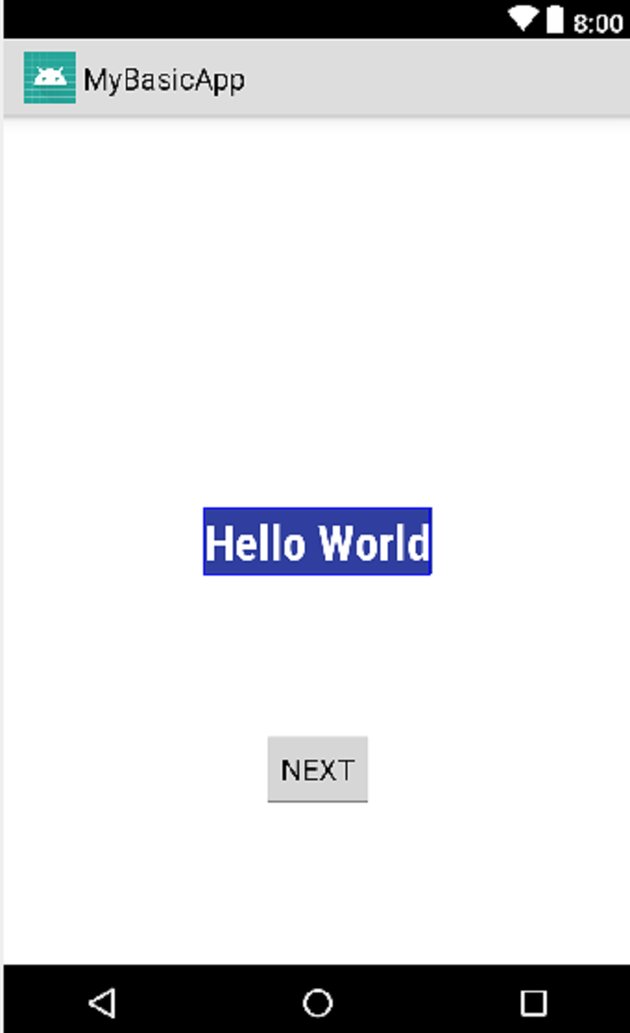
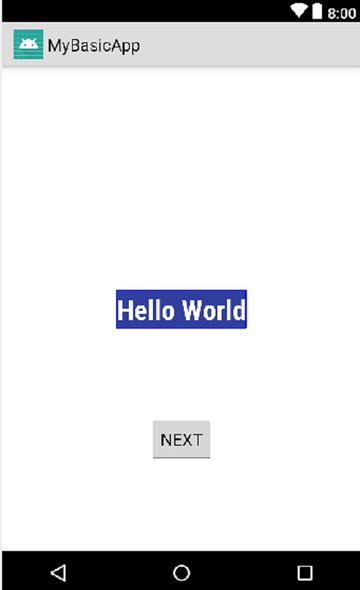
Here is the XML code for the finished layout:
<TextView android:id="@+id/textview_first" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@color/colorPrimaryDark" android:fontFamily="sans-serif-condensed" android:text="Hello World" android:textColor="@android:color/white" android:textSize="30sp" android:textStyle="bold" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" />
Assigning Activity to the Button
The layout design is the meat of your app. You have added a Button
, but it doesn’t do anything when pressed. To make it interactive and responsive to users’ actions, we have to assign activity to it.
Let’s say this is the last step on your app, and upon clicking Next you want people to see the message Finish.
Here’s how to do it..
The Next buttonhas an id called @+id/next
. Since no other element is using this id, we can use it to find the button and add it to the Java code so that the activity doesn’t get associated with another button.
The id for a view helps you identify it as each id differs from other viewers’ id.
With the findViewByID()
function, next
can be found through its id, R.id.next
.
Here is the final code that will display the finish message:
view.findViewById(R.id.next).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Toast myToast = Toast.makeText(MainActivity.this, "FINISH", Toast.LENGTH_SHORT); myToast.show(); } });
Run the app to see how the Button
works.
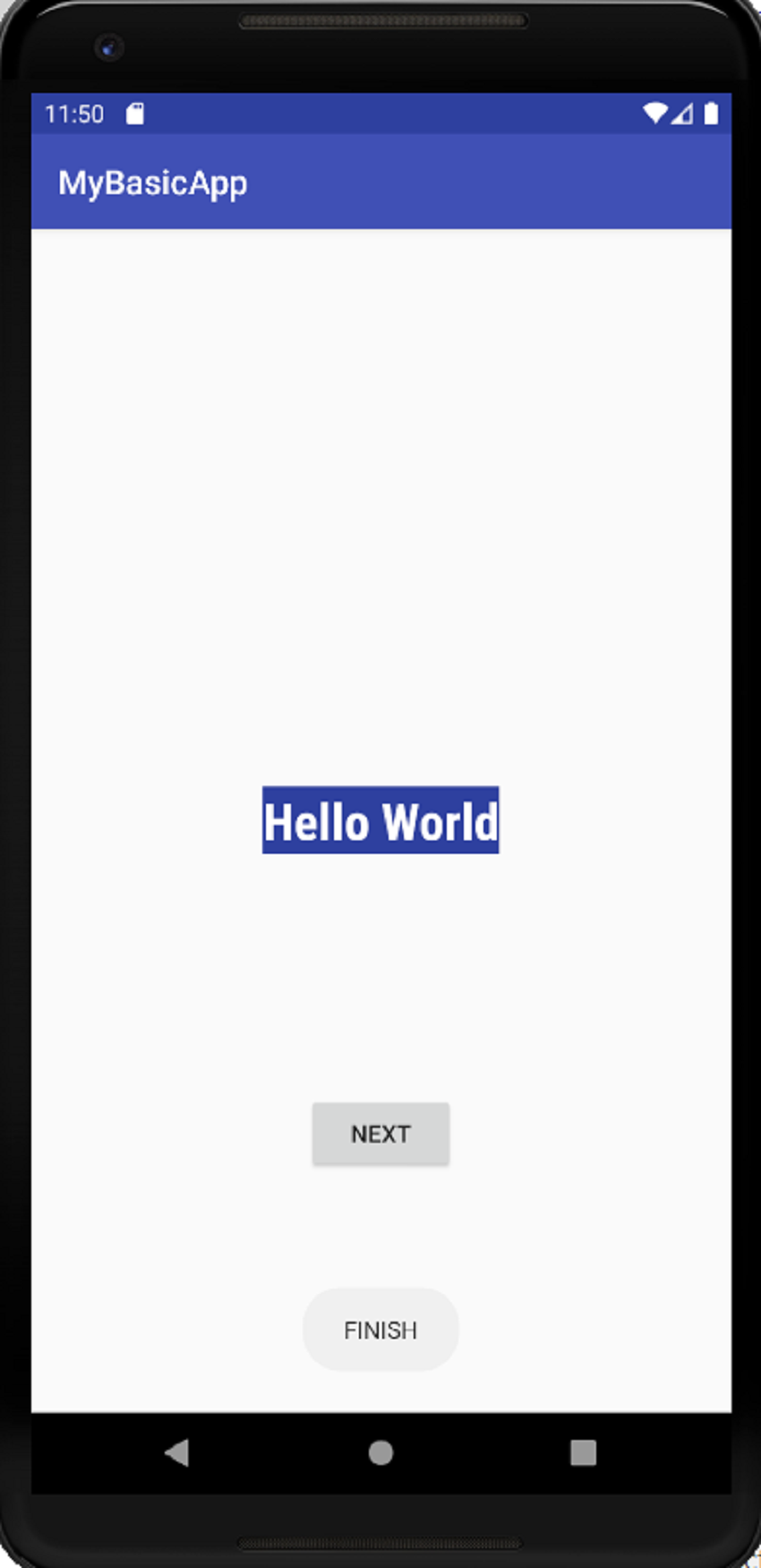
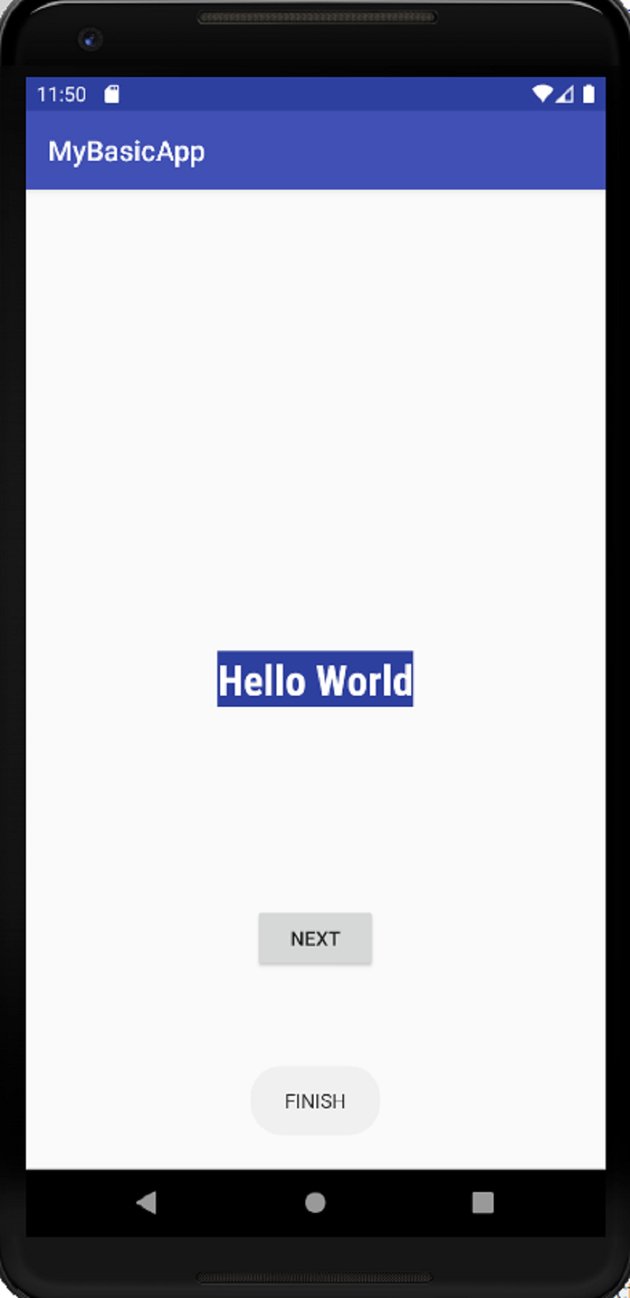
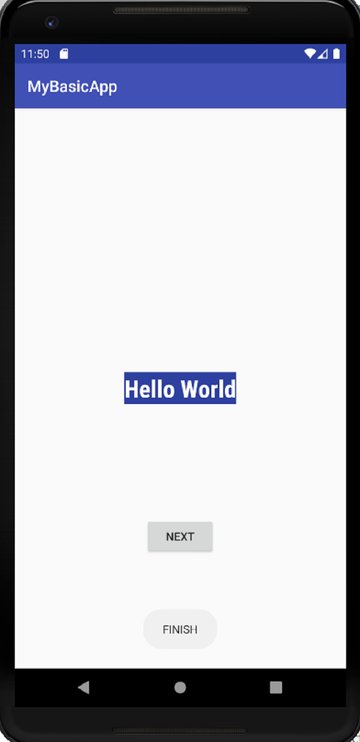
Conclusions
This guide covered everything from setting up the Android Studio to coding and testing a Button
. It’s not hard to get started coding an app—Java mobile app development has become easier since a lot of elements are drag and drop.
Android App Templates and UI Kits From CodeCanyon
You may have noticed that our app looks very plain and simple. That’s because we’re using the default theme, without applying any styles to our views. CodeCanyon is full of Android UI kits that offer beautiful, hand-crafted styles you can apply to your views.
The kits generally also have several custom views and layouts. You can refer to the following articles to learn more about them: