A webpage consists of a variety of HTML elements which are created using different tags. Some common tags include the <html>
tag which represents the root of an HTML document, the <body>
tag which represents the content of the document, the p
tag which represents paragraphs and so on.
Different tags that you use to create a webpage can also have different attributes attached to them. These attributes will either change the appearance or behavior of the elements or provide additional metadata. Some examples of attributes include id
, class
, contenteditable
, minlength
, maxlength
, and pattern
.
In this tutorial, we will learn how to manipulate different attributes of any element on a webpage.
Getting Attribute Values from Elements
We can call the getAttribute()
method on our element and pass it the name of our attribute to retrieve its value. The return value of this method is a string that represents the value of the attribute.
You will get back null
when the attribute whose value you are retrieving does not actually exist.
Let’s try to get the attribute values for the ordered list in the following markup:
1 |
<h1>Most Populated Countries</h1> |
2 |
|
3 |
<ol type="I" start="10" reversed="true"> |
4 |
<li>Mexico</li> |
5 |
<li>Russia</li> |
6 |
<li>Bangladesh</li> |
7 |
<li>Nigeria</li> |
8 |
<li>Brazil</li> |
9 |
</ol>
|
Here is the JavaScript that gets the value of all three attributes:
1 |
let pop_countries = document.querySelector("ol"); |
2 |
|
3 |
// Output: 10
|
4 |
console.log(pop_countries.getAttribute("start")); |
5 |
|
6 |
// Output: true
|
7 |
console.log(pop_countries.getAttribute("reversed")); |
8 |
|
9 |
// Output: I
|
10 |
console.log(pop_countries.getAttribute("type")); |
Setting Attribute Values for Elements
As I mentioned earlier, attributes are used to either change the behavior of an element or provide additional metadata. You might need to update the value of an existing attribute to something else or add a new attribute to an element.
You can use the setAttribute()
method in either case. It accepts two parameters—the attribute name and the attribute value. The attribute name has to be a string and it will be converted to all lowercase. The attribute value is also a string and any non-string values will be automatically converted to a string.
Consider the following markup:
1 |
<ol type="I" start="10" reversed="true"> |
2 |
<li>Brazil</li> |
3 |
<li>Nigeria</li> |
4 |
<li>Bangladesh</li> |
5 |
<li>Russia</li> |
6 |
<li>Mexico</li> |
7 |
</ol>
|
The following JavaScript will update the value of type
, start
and reversed
attributes in our list:
1 |
let pop_countries = document.querySelector("ol"); |
2 |
|
3 |
pop_countries.setAttribute("type", "i"); |
4 |
pop_countries.setAttribute("start", "6"); |
5 |
pop_countries.setAttribute("reversed", "false"); |
This results in the following markup:
1 |
<ol type="i" start="6" reversed="false"> |
2 |
<li>Brazil</li> |
3 |
<li>Nigeria</li> |
4 |
<li>Bangladesh</li> |
5 |
<li>Russia</li> |
6 |
<li>Mexico</li> |
7 |
</ol>
|
The following image shows how to order list was rendered originally and after the updates to attribute values.
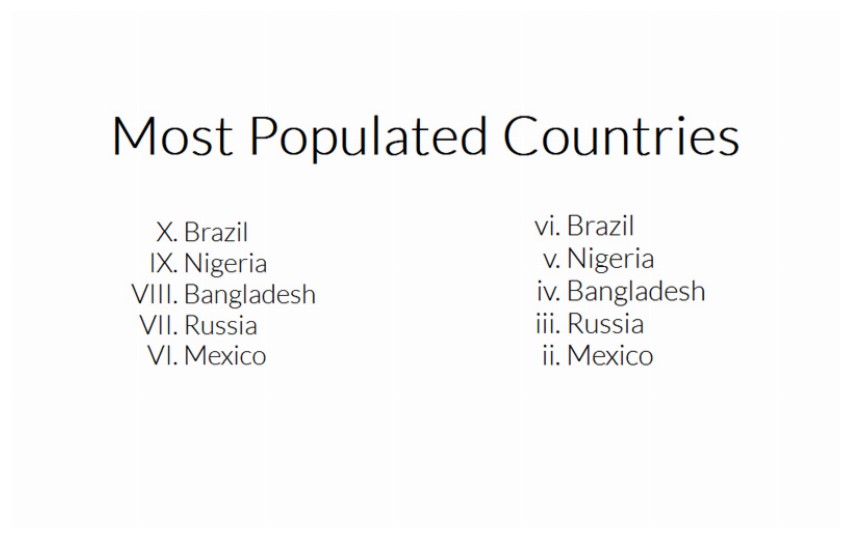
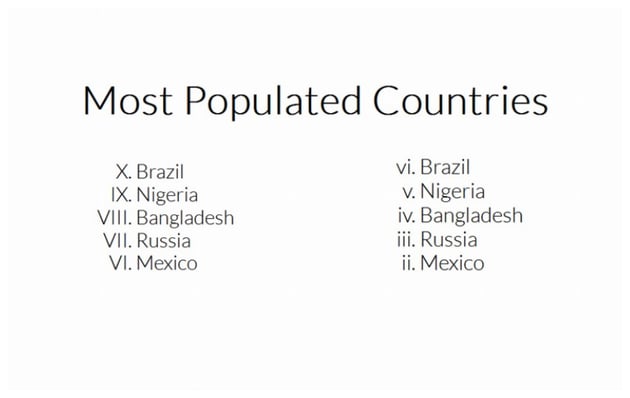
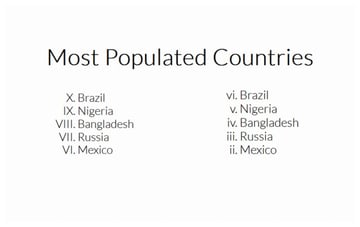
As you can see, the counting order is still reversed even though we have set the value of reversed
to false
. The reason this happens is because reversed
is a Boolean attribute and setting it to any value will result in its value being considered true.
It is recommended that you set the value of such attributes to either an empty string or attribute names if you want them to be true. The only way to prevent the reversal from happening is to remove the attribute entirely using the removeAttribute()
method.
1 |
pop_countries.removeAttribute("reversed"); |
Add, Remove, Replace or Toggle Element Classes
You probably already know about the class
attribute that we can use with different HTML elements to provide a space-separated list of classes. Classes play an important role in selection of elements for either applying some styling or scripting.
Adding, removing, replacing and toggling classes of an element is actually a very common task in web development. An element might need to be in active or inactive state which you can show by use of different classes.
Although we can use the getAttribute()
and setAttribute()
methods to do all these operations on an element’s classes, it is much more convenient to just use the read-only classList
property along with its add()
, remove()
, replace()
and toggle()
helper methods.
The add()
Method
The classList
property gives back a DOMTokenList
of classes attached to the queried element. You can use the add()
method to add new tokens or class names to the list. It is possible to pass multiple class names to this method and any existing token will not be added twice but simply omitted.
Here are some examples of using this method:
1 |
let message = document.querySelector(".message"); |
2 |
|
3 |
// Add a single class
|
4 |
message.classList.add("notification"); |
5 |
|
6 |
// Add multiple classes
|
7 |
message.classList.add("alert", "message"); |
The remove()
Method
You can use the remove()
method if you want to remove one or more classes from an element. No errors will be thrown if the class that you want to remove from an element is already absent from the element’s class list.
Here are some examples of using this method:
1 |
let message = document.querySelector(".message"); |
2 |
|
3 |
// Remove a single class
|
4 |
message.classList.remove("notification"); |
5 |
|
6 |
// Remove multiple classes
|
7 |
message.classList.remove("alert", "hidden"); |
The toggle()
Method
The toggle()
method is useful when you want to remove a class if it is in the DOMTokenList
and add a class if it is not in the DOMTokenList
. This method can accept either one or two parameters. The first parameter is the name of the class that you want to toggle. The second parameter is a condition that you want to check before you toggle the class. When the second parameter returns false
, the class can only be removed and not added. When the second parameter returns true
, the class can only be added and not removed.
This method returns true
when a class is added to the DOMTokenList
and returns false
when a class is removed from the DOMTokenList
. You can use the return value to confirm if the class is present or absent after the operation.
1 |
message.classList.toggle("hidden"); |
The replace()
Method
Some times, we want to replace an existing class with a new one and do nothing if the old class isn’t already attached to the element. The replace()
method works best in this case. It will return false
if the replacement failed and true
if the replacement was successful.
Here is some code that listens to the click event on a button and then takes all paragraphs with a particular class and replaces that class with a new one.
1 |
done_btn.addEventListener("click", (evt) => { |
2 |
let planned_tasks = document.querySelectorAll(".will-do"); |
3 |
|
4 |
for(task of planned_tasks) { |
5 |
task.classList.replace("will-do", "done"); |
6 |
}
|
7 |
});
|
The following CodePen shows all these four methods in action with the classes add, removed, toggled or replaced based on button clicks.
Final Thoughts
In this tutorial, we have learned about native JavaScript methods to add, update or remove attributes to different elements on your webpage. It is very common for developers to manipulate the classes associated with an element. JavaScript allows you to do it much more easily with the classList
property and its helper methods.