In the first part of this series about using CreateJs, we had a look at EaselJs. In this second and last part, we will look at PreloadJs, SoundJs, and TweenJs.
PreloadJS
PreloadJS is a library that lets you manage and co-ordinate the loading of assets. PreloadJS makes it easy to preload your assets, like images, sounds, JS, data, and others. It uses XHR2 to provide real progress information when available or fall back to tag-loading and eased progress when it is not. It allows multiple queues, multiple connections, pausing queues, and a lot more.
In PreloadJS, the LoadQueue class is the main API for preloading content. LoadQueue is a load manager, which can preload either a single file or a queue of files. var queue = new createjs.LoadQueue(true);
, passing false would force tag-loading where possible. LoadQueue has several events you can subscribe to:
- complete: fired when a queue completes loading all files
- error: fired when the queue encounters an error with any file.
- progress: the progress for when the entire queue has changed.
- fileload: for when a single file has completed loading.
- fileprogress: the progress for when a single file has changes. Please note that only files loaded with XHR (or possibly by plugins) will fire progress events, other than zero or 100%.
You can load single files by calling loadfile("path to file")
or load multiple files by calling loadManifest()
and passing in an array of the files you wish to load.
The supported file types of LoadQueue
include the following.
- BINARY: Raw binary data via XHR
- CSS: CSS files
- IMAGE: Common image formats
- JAVASCRIPT: JavaScript files
- JSON: JSON data
- JSONP: JSON files cross-domain
- MANIFEST: A list of files to load in JSON format, see loadManifest
- SOUND: Audio file formats
- SVG: SVG files
- TEXT: Text files – XHR only
- XML: XML data
Here is an example of using PreloadJS to load in a JavaScript file, some PNGs, and an MP3.
var canvas = document.getElementById("Canvas"); var stage = new createjs.Stage(canvas); var manifest; var preload; var progressText = new createjs.Text("", "20px Arial", "#000000"); progressText.x = 300 - progressText.getMeasuredWidth() / 2; progressText.y = 20; stage.addChild(progressText); stage.update(); function setupManifest() { manifest = [{ src: "collision.js", id: "myjsfile" }, { src: "logo.png", id: "logo" }, { src: "ForkedDeer.mp3", id: "mp3file" } ]; for(var i=1;i<=13;i++) manifest.push({src:"c"+i+".png"}) } function startPreload() { preload = new createjs.LoadQueue(true); preload.installPlugin(createjs.Sound); preload.on("fileload", handleFileLoad); preload.on("progress", handleFileProgress); preload.on("complete", loadComplete); preload.on("error", loadError); preload.loadManifest(manifest); } function handleFileLoad(event) { console.log("A file has loaded of type: " + event.item.type); if(event.item.id == "logo"){ console.log("Logo is loaded"); //create bitmap here } } function loadError(evt) { console.log("Error!",evt.text); } function handleFileProgress(event) { progressText.text = (preload.progress*100|0) + " % Loaded"; stage.update(); } function loadComplete(event) { console.log("Finished Loading Assets"); } setupManifest(); startPreload();
Within the setupManifest()
function we are adding a JavaScript file, an MP3, and a PNG file. We pass an object in with “src” and “id” keys. By using “id” we will be able to identify the asset once it has loaded. Lastly, we loop through and add 13 more images into the array. You won’t always need an “id” if you just want to make sure some assets get loaded.
We are listening for the “fileload”, “progress”, and “complete” events. The fileload event fires when a single file has loaded, progress is for getting the progress of the loadQueue, and complete fires when all the files have loaded. In the handleFileLoad()
function we are logging the files type, which can come in very handy. We also check the item’s id
, this is how you can keep track of the preloads and do something appropriate with the assets.
Note , that to be able to load sound, you must call installPlugin(createjs.Sound)
which we have done within the startPreload
function.
TweenJS
The TweenJS library is for tweening and animating HTML5 and JavaScript properties. It provides a simple but powerful tweening interface. It supports tweening of both numeric object properties and CSS style properties, and allows you to chain tweens and actions together to create complex sequences.
To setup a Tween, you call the Tween(target, [props], [pluginData])
constructor with the following options.
- target – The target object that will have its properties tweened
- props – The configuration properties to apply to this tween instance (for example, {loop:true, paused:true}). All properties default to false. Supported props are:
- loop: sets the loop property on this tween.
- useTicks: uses ticks for all durations instead of milliseconds.
- ignoreGlobalPause: sets the ignoreGlobalPause property on this tween.
- override: if true, Tween.removeTweens(target) will be called to remove any other tweens with the same target.
- paused: indicates whether to start the tween paused.
- position: indicates the initial position for this tween.
- onChange: specifies a listener for the “change” event.
- pluginData – An object containing data for use by installed plugins
The Tween class tweens properties for a single target. Instance methods can be chained for easy construction and sequencing. It is not a very big class and only has a few methods, but it is very powerful.
The to(props,duration,ease)
method, queues a tween from the current values to the target properties. Set duration to 0
to jump immediately to the value. Numeric properties will be tweened from their current value in the tween to the target value. Non-numeric properties will be set at the end of the specified duration.
The wait(duration,passive)
queues a wait (essentially an empty tween).
The call(callback,params,scope)
method Queues an action to call the specified function
TweenJS supports a large number of eases supplied by the Ease class.
In the following demo, you can click on the stage to see the demo.
In this demo, we create Bitmap
and Text
objects. We put the logo off the top of the stage and when the user clicks on the stage we tween it to the middle of the stage while changing its X and Y scale. We use the wait()
method to give a one second delay, then we use the call()
method to tween the text. In the text tween, we change the alpha, do a 360 degrees rotation, the tween it off to the left of the stage.
TweenJS is a lot of fun, try using some of the other properties of the display objects
SoundJS
SoundJS is a library that provides a simple API and powerful features to make working with audio a breeze. Most of this library is used in a static way (you do not need to create an instance). It works via plugins which abstract the actual audio implementation, so playback is possible on any platform without specific knowledge of what mechanisms are necessary to play sounds.
To use SoundJS, use the public API on the Sound class. This API is for:
- Installing audio playback plugins
- Registering (and preloading) sounds
- Creating and playing sounds
- Master volume, mute, and stop controls for all sounds at once
Playing sounds creates SoundInstance
instances, which can be controlled individually. You can:
- Pause, resume, seek, and stop sounds
- Control a sound’s volume, mute, and pan
- Listen for events on sound instances to get notified when they finish, loop, or fail
Below is the minimal code you will need to play an audio file.
createjs.Sound.initializeDefaultPlugins(); createjs.Sound.alternateExtensions = ["ogg"]; var myInstance = createjs.Sound.play("IntoxicatedRat.mp3");
I could not however, get that to work on my machine in Firefox and Chrome, although the above worked in IE. The default plugin was defaulting to WebAudio, I had to register it to use the HTMLAudio as below.
createjs.Sound.registerPlugins([ createjs.HTMLAudioPlugin]); console.log(createjs.Sound.activePlugin.toString()); createjs.Sound.alternateExtensions = ["ogg"]; var mySound = createjs.Sound.play("IntoxicatedRat.mp3");
In the above sections of code, we use the alternateExtensions
property which will attempt to load the file type OGG if it cannot load MP3.
With the very basics out of the way, we will now be creating an MP3 player. See the demo and screenshot below.
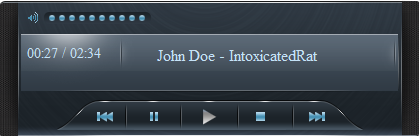
We first call registerPlugins()
to use HTMLAudio and set alternate extension so it will try to load OGG if the browser does not support MP3.
createjs.Sound.registerPlugins([ createjs.HTMLAudioPlugin]); createjs.Sound.alternateExtensions = ["ogg"];
Within the playSound()
function, we call the play()
method which plays one of the sound files from the tracks
array. We set the sounds volume by using the setVolume()
method, we use the “complete” listener to be alarmed when the current sound is finished playing.
theMP3 = createjs.Sound.play("./sounds/"+tracks[currentSong]+".mp3"); theMP3.setVolume(vol); theMP3.on("complete",songFinishedPlaying,null,false);
Throughout the rest of the code, we use the play()
, stop()
,pause()
and resume()
methods.
theMP3.play(); theMP3.stop(); theMP3.pause(); theMP3.resume();
I have not given a line by line explanation of the MP3 player, but if you have any questions, feel free to ask in the comments.
Space Invader Game
As mentioned in the first half of this tutorial, here is a demo of the Space Invaders game with preloading, sound, and tweens added in.
Conclusion
This concludes our tour of CreateJS. Hopefully from the past two articles, you have seen how easy it is to create rich interactive application with CreateJS.
The documentation is top notch and it comes with a huge amount of examples, so be sure to check them out.
I hope you have found this tutorial to be helpful and that you are excited about using CreateJS. They have just announced on their blog that beta support for WebGL is now available as well. Thanks for reading!