Anyone who has been writing code for a while knows how important it is to write code that is optimized. I am sure you have encountered situations where you wrote a script that was taking a long time to execute and wanted to optimize it so that it ran faster.
Optimization doesn’t always have to mean that the code executes faster. You might also want to optimize for memory usage if you are short on memory. Similarly, you might want to optimize for usage of any other resources, code maintainability, etc.
In this tutorial, I will show you how to use ChatGPT to optimize your code.
Why Optimize Code?
There are several reasons why you might want to optimize code. The obvious one is improved performance. Any script that executes quickly will result in cost savings for you, as well as a better experience for your users.
Optimized code is also going to be scalable. For example, I once wrote some code that kept instantiating a new object until the task at hand was done. This would sometimes result in “out of memory” errors.
One solution was just to increase the memory limit. It worked for a while. However, I was out of memory once again when I was trying to solve bigger problems. Inefficient code is going to run out of resources sooner or later.
In my case, I rewrote the code and ended up with a memory usage of a few MB instead of 4GB.
Using ChatGPT for Code Optimization
In the past, we had to rely on other people from websites like Stack Overflow to help us optimize our code and solve any other issues that we might be facing. There is no denying that Stack Overflow and other such communities are still invaluable when you want to get figure out how to solve a complicated problem. However, you can now also start using ChatGPT to gain some insight into your problems and optimize the code.
One of its biggest advantages is that ChatGPT will give you an instant response. It has also been trained on a large amount of data, so it has a decent knowledge of programming concepts and best practices to follow.
Optimizing the Code for Speed
In this section, you will learn how we can optimize our code for speed using ChatGPT. We will use Problem 10 from Project Euler as our example. It states that:
The sum of the primes below 10 is 2+3+5+7=17.
Find the sum of all the primes below two million.
Looking at the problem, we know that we need to find all the primes below 2 million before we can calculate their sum. We will create an array to store the primes. We will also need to use two for
loops. The outer one will iterate from 2 to 2 million. The inner loop will check if any of those numbers are prime.
Here is the code that I have written to do this in JavaScript:
1 |
let primes = []; |
2 |
|
3 |
function find_primes_in_range(min, max) { |
4 |
for(let i = min; i <= max; i++) { |
5 |
let is_prime = true; |
6 |
for(let j = 2; j <= i/2; j++) { |
7 |
if(i%j == 0) { |
8 |
is_prime = false; |
9 |
break; |
10 |
}
|
11 |
}
|
12 |
|
13 |
if(is_prime) { |
14 |
primes.push(i); |
15 |
}
|
16 |
}
|
17 |
}
|
18 |
|
19 |
let start = Date.now(); |
20 |
|
21 |
find_primes_in_range(2, 2000000); |
22 |
|
23 |
let sum = 0; |
24 |
|
25 |
for(let i = 0; i < primes.length; i++) { |
26 |
sum += primes[i]; |
27 |
}
|
28 |
|
29 |
// Output: Sum of Primes: 142913828922
|
30 |
console.log(`Sum of Primes: ${sum}`); |
31 |
|
32 |
let end = Date.now(); |
33 |
|
34 |
let seconds = (end - start)/1000; |
35 |
|
36 |
// Output: Total Time: 201.489
|
37 |
console.log(`Total Time: ${seconds}`); |
Our algorithm for calculating the sum of primes is rather primitive and takes over 200 seconds. Let’s see if ChatGPT can improve it in any way.
Add the following prompt in ChatGPT, and then place your code below the prompt:
1 |
How can I optimize the JavaScript code below so that it runs quickly? It is supposed to calculate the sum of all primes below two million. |
ChatGPT suggests two things that we can do to make sure our code executes faster.
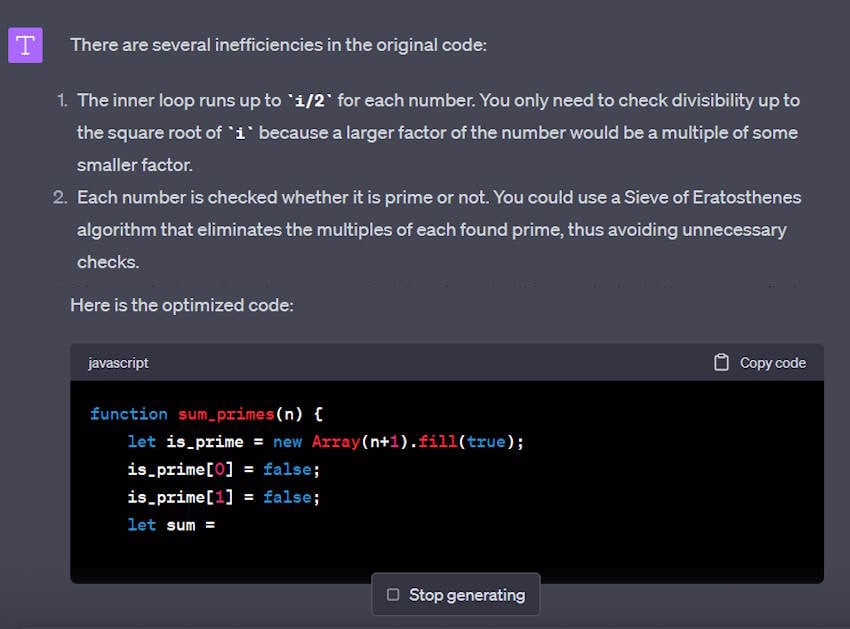
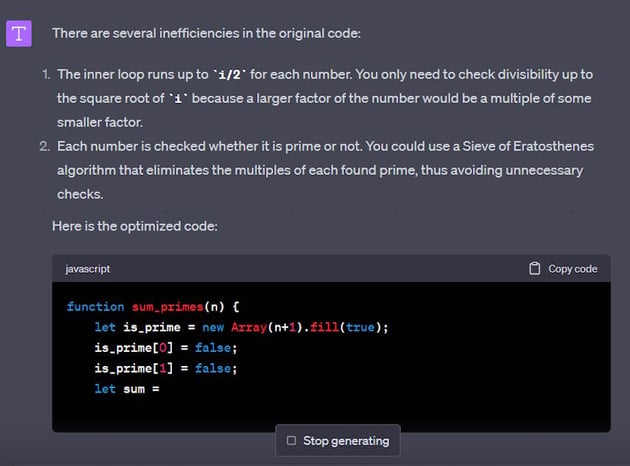
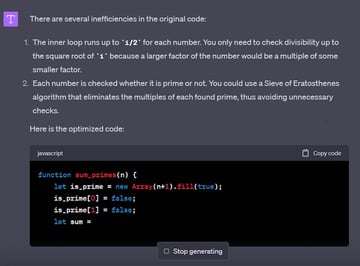
First, it tells us to optimize the prime-checking algorithm so that we only iterate up to Math.sqrt(i)
in the inner loop. Second, it advocates for the use of the Sieve of Eratosthenes. It works by marking all the multiples of a prime number as a composite. The only numbers left at the end are primes.
1 |
function sumPrimesBelow(n) { |
2 |
let primes = []; |
3 |
let isPrime = Array(n).fill(true); |
4 |
let sum = 0; |
5 |
|
6 |
for (let i = 2; i < n; i++) { |
7 |
if (isPrime[i]) { |
8 |
primes.push(i); |
9 |
sum += i; |
10 |
|
11 |
for (let j = i * i; j < n; j += i) { |
12 |
isPrime[j] = false; |
13 |
}
|
14 |
}
|
15 |
}
|
16 |
|
17 |
return sum; |
18 |
}
|
19 |
|
20 |
let start = Date.now(); |
21 |
let sum = sumPrimesBelow(2000000); |
22 |
let end = Date.now(); |
23 |
|
24 |
// Output: Sum of Primes: 142913828922
|
25 |
console.log(`Sum of Primes: ${sum}`); |
26 |
|
27 |
// Output: Total Time: 0.049 seconds
|
28 |
console.log(`Total Time: ${(end - start) / 1000} seconds`); |
The running time of our script has improved significantly. It is around 4,000 times faster.
Optimizing the Code for Memory
Our original version of the code to calculate the sum of primes stored them all in an array, which consumes memory. We can avoid creating an array by simply calculating the running sum of the primes. As soon as we know that a number is prime, we add it to our current sum.
1 |
Can you optimize this JavaScript code so that it uses the least possible amount of memory? |
Here is the output that I received:
1 |
function sumPrimesBelow(n) { |
2 |
let sum = 0; |
3 |
|
4 |
for (let i = 2; i < n; i++) { |
5 |
let isPrime = true; |
6 |
|
7 |
for (let j = 2; j <= Math.sqrt(i); j++) { |
8 |
if (i % j === 0) { |
9 |
isPrime = false; |
10 |
break; |
11 |
}
|
12 |
}
|
13 |
|
14 |
if (isPrime) { |
15 |
sum += i; |
16 |
}
|
17 |
}
|
18 |
|
19 |
return sum; |
20 |
}
|
21 |
|
22 |
let start = Date.now(); |
23 |
let sum = sumPrimesBelow(2000000); |
24 |
let end = Date.now(); |
25 |
|
26 |
// Output: Sum of Primes: 142913828922
|
27 |
console.log(`Sum of Primes: ${sum}`); |
28 |
|
29 |
// Output: Total Time: 0.53 seconds
|
30 |
console.log(`Total Time: ${(end - start) / 1000} seconds`); |
This time, we have traded some of our speed for less memory usage. The script now takes 0.5 seconds to execute, which is 10 times slower than using the Sieve of Eratosthenes. However, it is still 400 times faster than our original script.
Making Code Shorter and More Readable
In my previous tutorial, we discussed how you can use ChatGPT to learn coding quickly. In one section, we asked ChatGPT to generate code that uses both lists and for
loops. It gave us the following code in response:
1 |
numbers = [1, 2, 3, 4, 5] |
2 |
|
3 |
# Compute the square of each number in the list using a for loop
|
4 |
squared_numbers = [] |
5 |
for num in numbers: |
6 |
squared_numbers.append(num ** 2) |
7 |
|
8 |
# Print the squared numbers
|
9 |
for squared_num in squared_numbers: |
10 |
print(squared_num) |
Usually, there are multiple ways of writing a piece of code to achieve the same functionality. Sometimes, there are language-specific features that can make code that you have written shorter while maintaining or improving readability.
For example, the following prompt asks ChatGPT to make the given Python code shorter:
1 |
Can I make this Python code shorter while maintaining readability? |
The response that I get back is:
1 |
numbers = [1, 2, 3, 4, 5] |
2 |
|
3 |
# Compute the square of each number using a list comprehension
|
4 |
squared_numbers = [num ** 2 for num in numbers] |
5 |
|
6 |
# Print the squared numbers using a for loop
|
7 |
for squared_num in squared_numbers: |
8 |
print(squared_num) |
Let’s say you had written the same code in JavaScript. It would look something like this:
1 |
let numbers = [1, 2, 3, 4, 5]; |
2 |
|
3 |
let squared_numbers = []; |
4 |
for (let i = 0; i < numbers.length; i++) { |
5 |
squared_numbers.push(numbers[i] ** 2); |
6 |
}
|
7 |
|
8 |
for (let i = 0; i < squared_numbers.length; i++) { |
9 |
console.log(squared_numbers[i]); |
10 |
}
|
You could ask ChatGPT to use ES6 to make your code shorter, while maintaining readability, by using the following prompt:
1 |
Make this JavaScript code shorter using ES6 while maintaining readability. |
In my case, the output was:
1 |
let numbers = [1, 2, 3, 4, 5]; |
2 |
|
3 |
let squared_numbers = numbers.map(num => num ** 2); |
4 |
|
5 |
squared_numbers.forEach(num => console.log(num)); |
ChatGPT also provides an explanation, as you can see in the screenshot below:
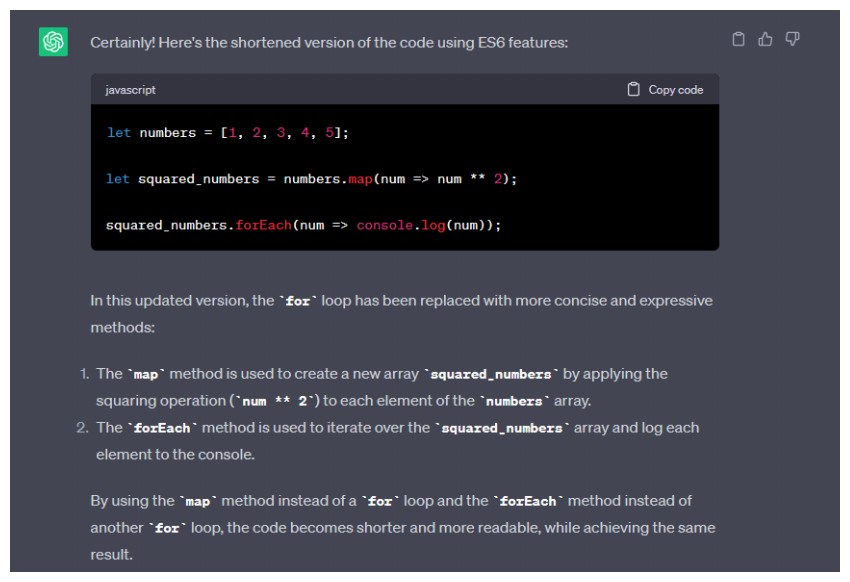
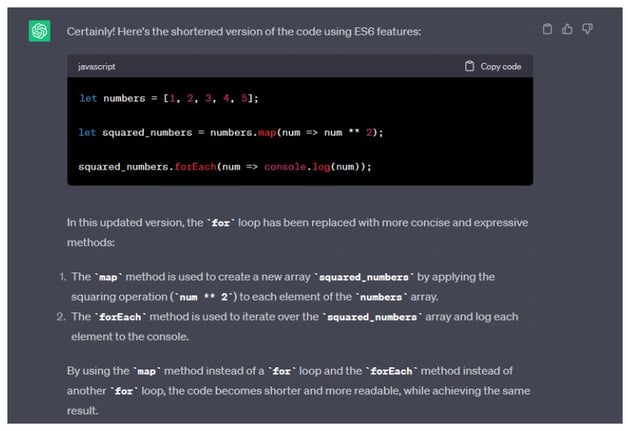
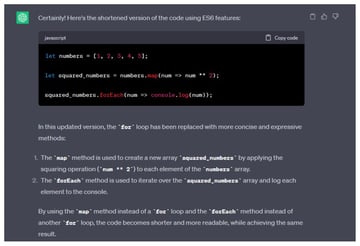
Final Thoughts
In this tutorial, we learned how to use ChatGPT to optimize code written in different languages. Optimization isn’t just limited to speed and memory usage. You can also use ChatGPT to make your code more readable, as we did in the last section.
My suggestion would be that you ask ChatGPT for insights to learn how to do the same thing using a different approach. You can also explicitly ask ChatGPT to follow best practices while optimizing your code.