CSS anchor positioning promises to revolutionize how we design and position elements on the web. In this article, we’ll explore the current state of CSS positioning and compare it with the exciting new possibilities offered by CSS anchoring.
We’ll dive into real-world examples and code snippets to showcase the potential of this upcoming feature. Finally, we’ll discuss some limitations developers should know when utilizing CSS anchoring.
CSS Anchoring: A New Paradigm in Web Layouts
CSS anchoring provides a fresh approach to positioning and layout by introducing a set of properties and values that enable elements to be tethered to one another. It offers more flexibility, control, and simplicity than traditional CSS positioning.
One of the key features of CSS anchoring is the ability to pin an element to a specific position within its containing element. This allows for dynamic and responsive layouts without the need for complex calculations. Let’s take a look at an example:
1 |
.parent { |
2 |
position: relative; |
3 |
}
|
4 |
|
5 |
.child { |
6 |
position: absolute; |
7 |
top: 50%; |
8 |
left: 50%; |
9 |
transform: translate(-50%, -50%); |
10 |
anchor-position: center; |
11 |
}
|
In the above code snippet, the .child
element is positioned at the center of its parent element, regardless of the parent’s dimensions. The anchor-position
property provides a more intuitive and declarative way of achieving such layouts.
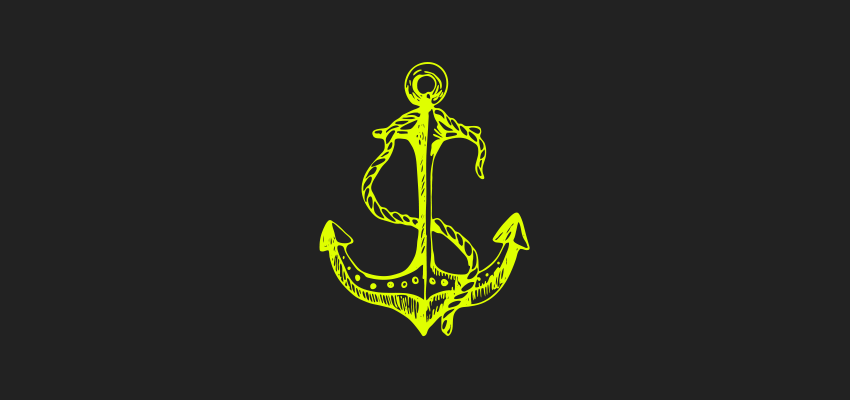
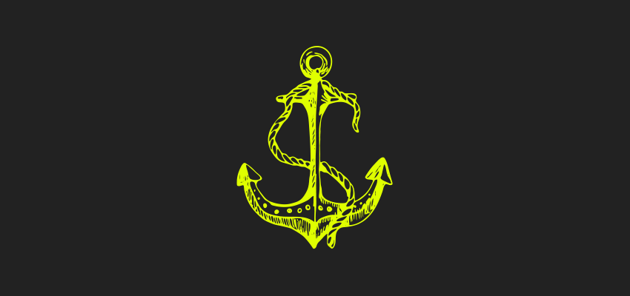
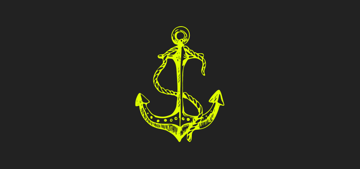
Defining an Anchor
To utilize CSS anchoring, you need to define an anchor that can be used to position other elements. Two ways to define an anchor are within your CSS or your HTML.
Defining an Anchor with CSS
First, you can define an anchor within your CSS by setting the anchor-name
property on the anchor element. It accepts a dashed-ident value.
1 |
.anchor { |
2 |
anchor-name: --my-anchor; |
3 |
}
|
In the code above, the .anchor
class defines an anchor named --my-anchor
.
Defining an Anchor with HTML
Alternatively, you can define an anchor in your HTML using the anchor
attribute. The attribute value is the ID
of the anchor element, creating an implicit anchor.
1 |
<a id="my-anchor" class="anchor"></a> |
2 |
|
3 |
<div anchor="my-anchor" class="bear">I’m a bear! </div> |
The code above defines an anchor using an empty <a>
element with the ID my-anchor
and the anchor
class. The div
element with the class boat
uses the anchor
attribute to specify that it should be tethered to the my-anchor
anchor.
Using the Anchor Function
Once you’ve defined an anchor, you can use the anchor function to position elements relative to it. The anchor function takes three arguments:
-
Anchor element: The anchor name of the anchor to use. If you omit the value, an implicit anchor will be used. An implicit anchor can be defined via the HTML relationship or with the
anchor-default
property using an anchor name value. -
Anchor side: A keyword or percentage value representing the position you want to use. This could be
top
,right
,bottom
,left
,center
, or a percentage. For example,50%
would represent the center position. - Fallback: An optional fallback value that accepts a length or percentage. The fallback value will be used if the anchor position is not supported or cannot be determined.
Here’s an example of using the anchor function:
1 |
.child { |
2 |
position: absolute; |
3 |
anchor: --my-anchor center; |
4 |
}
|
In the code above, the .child
element is positioned absolutely using the anchor
function, which specifies that it should be tethered to the --my-anchor
anchor at the center position.
Additional CSS Anchoring Features
In addition to the examples above, the draft specification for CSS anchoring introduces several more features that expand the possibilities of web layouts.
Anchor Edges
The CSS anchoring specification introduces the concept of anchor edges, which allows developers to define the exact edges to which an element should be anchored. This provides precise control over positioning. For example:
1 |
.element { |
2 |
anchor-edge: left top; |
3 |
}
|
The .element
is anchored to the left top corner of its containing element.
Anchor Margins
CSS anchoring also introduces the anchor-margin
property, which allows developers to specify additional margin around the anchored element. This is particularly useful when dealing with overlapping elements. For example:
1 |
.element { |
2 |
anchor-position: center; |
3 |
anchor-margin: 20px; |
4 |
}
|
Here the .element
is anchored at the center of its containing element, with an additional margin of 20 pixels.
Scroll Anchoring
Scroll anchoring is a feature that helps maintain the scroll position when elements are dynamically added or removed from the document. It prevents the page from jumping and provides a smoother scrolling experience for users. The scroll anchoring behavior is enabled by default, but it can be disabled if necessary:
1 |
html { |
2 |
overflow-anchor: none; |
3 |
}
|
The code above disables the scroll anchoring behavior for the entire HTML document.
Example
In this example, we’ll recreate a tooltip using CSS anchors rather than the older positioning method.
1 |
<div class="container"> |
2 |
|
3 |
<a id="my-anchor" class="anchor"> |
4 |
<svg xmlns=" https://www.w3.org/2000/svg" width= "24" height= "24" viewBox= "0 0 24 24"><title>information-circle</title><g fill= "none"><path d= "M11.25 11.25l.041-.02a.75.75 0 0 1 1.063.852l-.708 2.836a.75.75 0 0 0 1.063.853l.041-.021M21 12a9 9 0 1 1-18 0 9 9 0 0 1 18 0zm-9-3.75h.008v.008H12V8.25z" stroke-width= "1.5" stroke-linecap=" round" stroke-linejoin=" round"></path></g></svg> |
5 |
<span>This is some important notice in your application.</span> |
6 |
</a>
|
7 |
|
8 |
<div class="tooltip"> |
9 |
<h3>Tooltip</h3> |
10 |
<p>Tooltip content</p> |
11 |
</div>
|
12 |
</div>
|
The main change is how we approach CSS. The .anchor
class receives a new anchor-name
called tooltip, which allows us to define its anchoring neighbor with .tooltip
1 |
/* As of June 2023 this requires Chrome Canary with Experimental features enabled */
|
2 |
|
3 |
body { |
4 |
font-family: sans-serif; |
5 |
padding: 1rem; |
6 |
}
|
7 |
|
8 |
.container { |
9 |
margin: 4rem auto; |
10 |
max-width: 600px; |
11 |
}
|
12 |
|
13 |
.anchor { |
14 |
anchor-name: --tooltip; |
15 |
color: #444; |
16 |
font-weight: 500; |
17 |
padding: .5rem 1rem; |
18 |
border-top-left-radius: 6px; |
19 |
border-top-right-radius: 6px; |
20 |
}
|
21 |
|
22 |
.anchor svg { |
23 |
stroke: currentcolor; |
24 |
color: #444; |
25 |
width: 24px; |
26 |
height: 24px; |
27 |
position: relative; |
28 |
top: 6px; |
29 |
}
|
30 |
|
31 |
.anchor:hover ~ .tooltip { |
32 |
opacity: 1; |
33 |
visibility: visible; |
34 |
transition: ease-in .2s all; |
35 |
}
|
36 |
|
37 |
.tooltip { |
38 |
opacity: 0; |
39 |
visibility: hidden; |
40 |
position: fixed; |
41 |
|
42 |
anchor-default: --tooltip; |
43 |
bottom: anchor(auto); |
44 |
|
45 |
width: 400px; |
46 |
background: rgba(0, 0, 0, .01); |
47 |
padding: 1rem; |
48 |
border: 1px solid #ddd; |
49 |
border-radius: 6px; |
50 |
color: #333; |
51 |
transition: ease-in .2s all; |
52 |
}
|
53 |
|
54 |
.tooltip h3 { |
55 |
margin: 0; |
56 |
}
|
You can establish that relationship and then add additional styles and settings to construct the ideal positioning favored. Here the bottom style gets anchor(auto)
, which shifts the tooltip to the bottom of the anchored element. Adjust this with more properties like top
,right
,bottom
, left
, auto-same
.
auto-same
is equivalent to:
1 |
.cover { |
2 |
top: anchor(top); |
3 |
right: anchor(right); |
4 |
bottom: anchor(bottom); |
5 |
left: anchor(left); |
6 |
}
|
Limitations: What to Keep in Mind
While CSS anchoring opens up exciting possibilities for web layouts, it’s essential to be aware of its limitations. At the time of writing this article, the feature is still a draft, and browser support may vary. It’s crucial to test thoroughly and consider fallback options for unsupported browsers.
CSS anchoring may introduce complexities when working with complex or nested layouts. Elements with multiple anchoring relationships can create unexpected behaviors, and overlapping or intersecting anchors require additional consideration to achieve the desired results.
Conclusion
CSS anchoring presents an exciting new paradigm in web layout design, empowering developers to achieve complex layouts more quickly and efficiently. By tethering elements to each other, CSS anchoring eliminates the need for cumbersome workarounds and calculations, simplifying responsive and dynamic web designs.
As the CSS anchoring feature evolves, staying updated with the latest specifications and browser support is important. While it offers excellent potential, it’s also crucial to consider its current limitations and plan accordingly. CSS anchoring is set to redefine how we approach web layouts, providing a more intuitive and powerful tool for developers to create exceptional user experiences.