If you’re finding it difficult to understand and work with multidimensional arrays or nested arrays in JavaScript, then you’ve arrived at the right place. In this article, we’ll dive into nested arrays and go over the methods for updating, adding and removing items in a multidimensional array.
JavaScript Arrays
A JavaScript array is a collection of data that is stored in a list-like structure. Arrays are indexed — this means that each item in the array can be accessed using an index, which is an integer number starting from 0.
For example, a simple array could look like this:
1 |
let myArray = [1, 2, 3, 4, 5]; |
In this example, the first item in the array has an index of 0, the second item has an index of 1, and so on.
Arrays are most commonly used to store collections of data that need to be accessed quickly and easily. For example, if you have a list of users in your application, you could store them in an array and access them by their index.
1 |
let users = ["John", "Jane", "Jack"]; |
2 |
|
3 |
console.log(users[0]); // Outputs: "John" |
They can be used to store all kinds of values, including strings, numbers, and objects. It’s perfectly valid to store these different types of value within the same array.
1 |
const varied = [null, undefined, NaN, "string", 12345, {name: "Sam"}] |
You can also store (or nest) an array inside another array. This creates what is known as a multidimensional array.
Nested Arrays in JavaScript
A multidimensional array (also known as an array of arrays), is simply an array with one or multiple children arrays. These children arrays are said to be nested in the parent array.
1 |
const arrayOfArr = [ |
2 |
['value 1', 'value 2'] |
3 |
['value 3', 'value 4'] |
4 |
['value 5', 'value 6'] |
5 |
]
|
Nested arrays can be used to group together related elements. For example, take a look at the following multidimensional array:
1 |
const animalPairs = [ |
2 |
// female and male
|
3 |
['doe', 'buck'], |
4 |
['ewe', 'ram'], |
5 |
['peahen', 'peacock'], |
6 |
['cow', 'bull'], |
7 |
]
|
Here we’re pairing up the male and female names of the same animal in each nested array. Females are to the left and males to the right.
To access the first element, you’d use the index of 0 like so:
Prints the result on the console:
1 |
console.log(animalPairs[0]) |
2 |
|
3 |
// ['doe', 'buck'],
|
Now, to access a value from within a nested array, you have to add a second bracket containing the index of the value you want to access. For instance, let’s say you wanted to get the female name from the first array. Since you know that the female is always placed first, you simply:
- use
[0]
to get the first nested array, - then chain another
[0]
to get the first element inside that nested array (in our case, the female name):
Here’s the output:
1 |
console.log(animalPairs[0][0]) |
2 |
|
3 |
// 'doe'
|
If you want to get the second item in the first nested array:
1 |
animalPairs[0][1] // 'buck' |
In this case, we only have two items in each nested array, so our index terminates at [1]
. If you were to add more items, you can access each of them by their index.
To summarize, the first [ ]
returns the nested array while the second [ ]
returns a value from within that nested array.
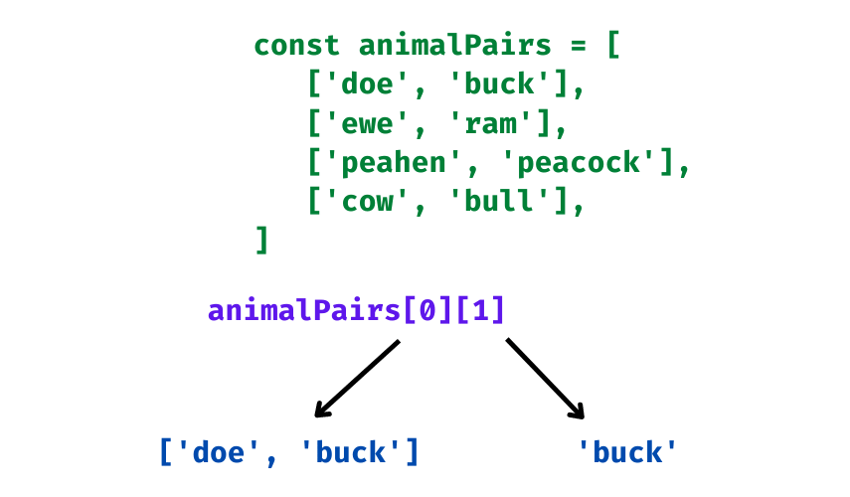
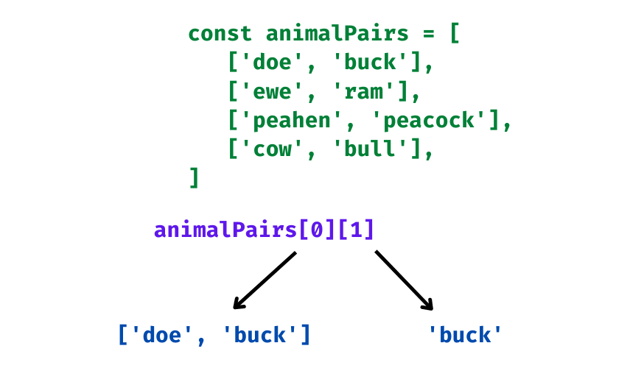
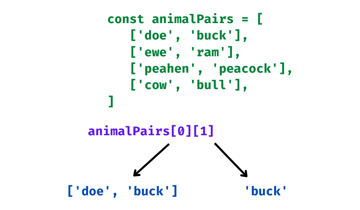
Updating Elements in the Nested Array
Let’s say that I was wrong about the first item, and the name of a male rabbit is stag and not buck. I can easily update the array by accessing the index and reassigning “stag” to it, like so:
1 |
animalPairs[0][1] = 'stag' |
Output:
1 |
console.log(animalPairs[0]) |
2 |
|
3 |
// ['doe', 'stag'],
|
You can also reassign a whole array (and not just one of its values) to a particular position in the main array using the index. This example changes the first nested array from rabbit to chicken:
1 |
animalPairs[0] = ['hen', 'rooster'] |
Adding and Removing Nested Arrays
You can nest a new array at the beginning and ending of another array, and you can remove an existing array from the start and end position of another array.
To nest an array at the beginning of the main one, pass the array to unshift() method:
1 |
const animalPairs = [ |
2 |
// hen and rooster will be inserted here
|
3 |
['doe', 'buck'], |
4 |
['ewe', 'ram'], |
5 |
['peahen', 'peacock'], |
6 |
['cow', 'bull'], |
7 |
]
|
8 |
|
9 |
animalPairs.unshift(['hen', 'rooster']) |
To insert an array at the end of the parent, pass the array to push() method:
1 |
const animalPairs = [ |
2 |
['doe', 'buck'], |
3 |
['ewe', 'ram'], |
4 |
['peahen', 'peacock'], |
5 |
['cow', 'bull'], |
6 |
// hen and rooster will be inserted here
|
7 |
]
|
8 |
|
9 |
animalPairs.push(['hen', 'rooster']) |
To remove the first nested array, simply execute the shift() method on the parent array:
1 |
const animalPairs = [ |
2 |
['doe', 'buck'], // removes this one |
3 |
['ewe', 'ram'], |
4 |
['peahen', 'peacock'], |
5 |
['cow', 'bull'], |
6 |
]
|
7 |
|
8 |
animalPairs.shift() |
Finally, to remove the last array, execute the pop() method on the parent array:
1 |
const animalPairs = [ |
2 |
['doe', 'buck'], |
3 |
['ewe', 'ram'], |
4 |
['peahen', 'peacock'], |
5 |
['cow', 'bull'], // removes this one |
6 |
]
|
7 |
|
8 |
animalPairs.pop() |
Conclusion
Using nested arrays is less common in real-world use cases. It’s much more common to nest multiple objects in an array (e.g., an array of user objects, each with properties like id
, username
, and so on)
However, knowing how to access and manipulate data in nested arrays helps you understand the language better, and also assists you in mastering the syntax for traversing complex array structures in JavaScript.