By default, WooCommerce allows us to exclude products that belong to specific categories from discount offers or to apply discounts only to products that belong to particular categories.
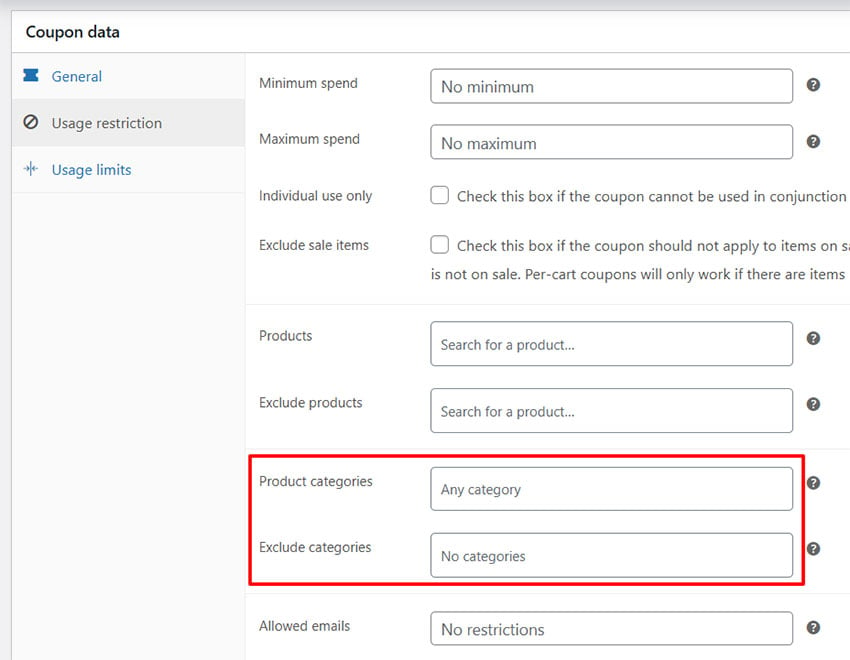
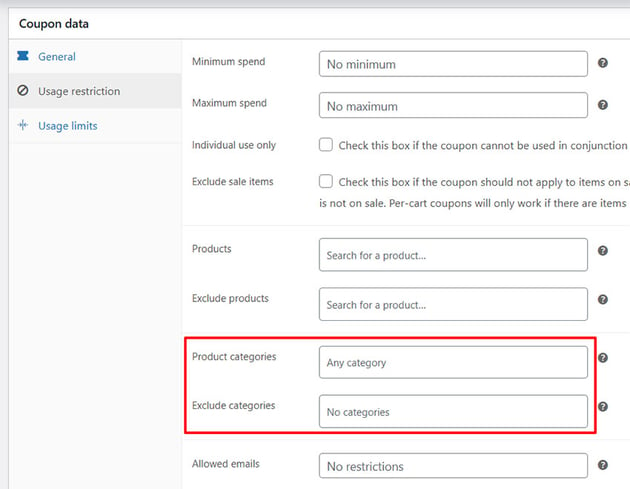
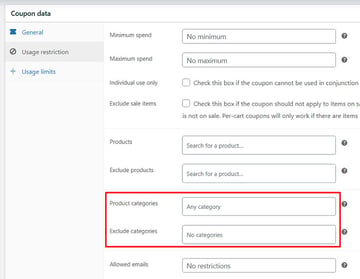
However, it doesn’t provide a straightforward mechanism for defining discount rules for other product taxonomies (e.g. brands and tags).
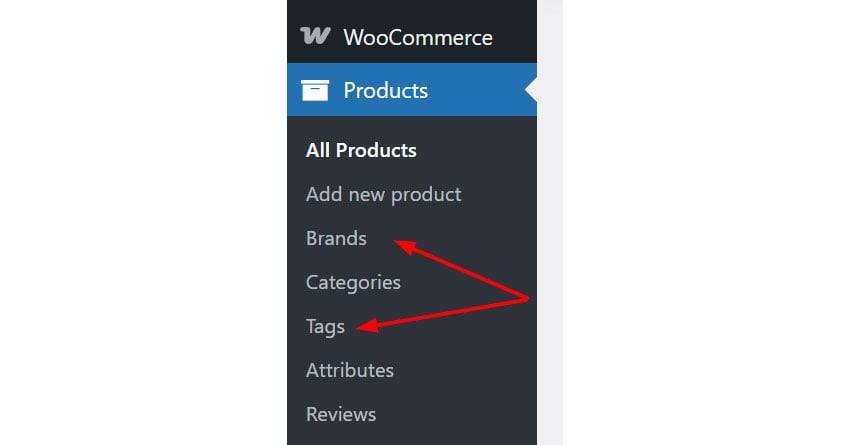
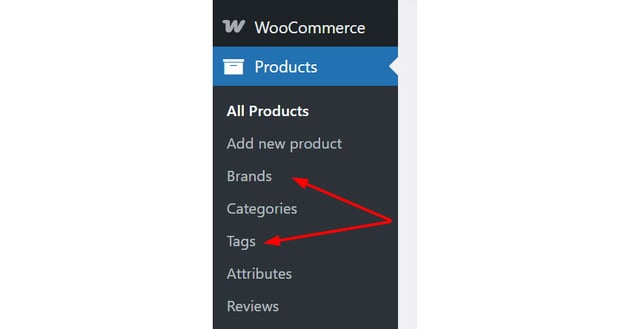
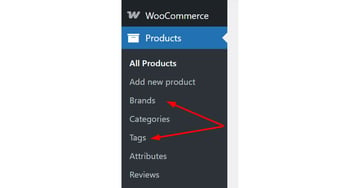
Of course, we can use a plugin to implement that feature, but it isn’t that difficult to implement ourselves!
All we have to do is take advantage of the woocommerce_coupon_is_valid_for_product
filter. If you want to dig deeper into it, you’ll find it inside the class-wc-coupon.php
file of the WooCommerce plugin files.
Let’s get a better understanding through some examples!
1. Set a discount only for products (simple or variable) without the Stock Offers tag.
To enable this rule, add the following code in the functions.php
file of your theme:
1 |
<?php
|
2 |
function wc_custom_coupon_rules( $valid, $product, $coupon, $values ) { |
3 |
$product_id = $product->get_ID(); |
4 |
if ( 'variation' === $product->get_type() ) : |
5 |
$product_id = $product->get_parent_id(); |
6 |
endif; |
7 |
if ( has_term( 'stock-offers', 'product_tag', $product_id ) ) : |
8 |
$valid = false; |
9 |
endif; |
10 |
return $valid; |
11 |
}
|
12 |
add_filter( 'woocommerce_coupon_is_valid_for_product', 'wc_custom_coupon_rules', 10, 4 ); |
With this rule in place, assuming we have available a welcome10 coupon for a 10% discount, a customer with these selections will enjoy a 2.50€ discount.
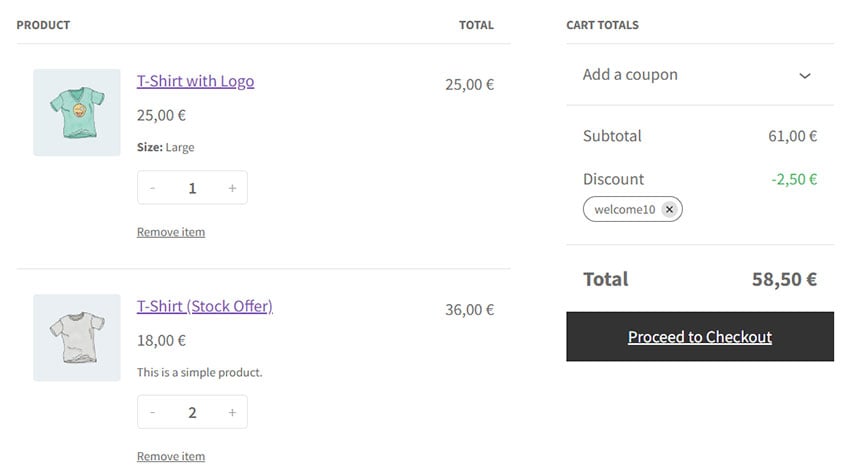
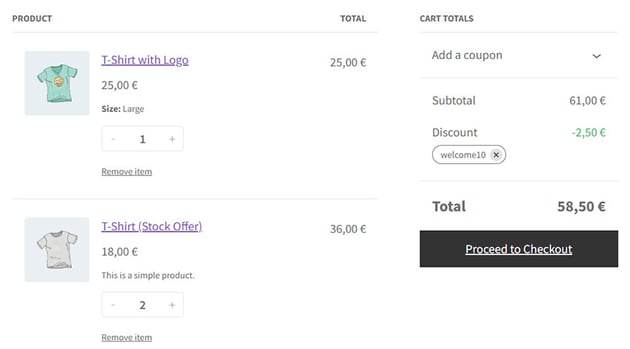
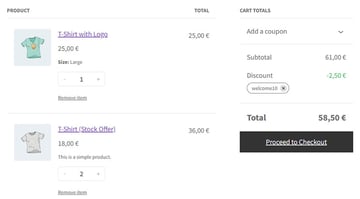
2. Set a discount only for products (simple or variable) with the Stock Offers tag.
To enable this rule, add the following code in the functions.php
file of your theme:
1 |
<?php
|
2 |
function wc_custom_coupon_rules( $valid, $product, $coupon, $values ) { |
3 |
$product_id = $product->get_ID(); |
4 |
if ( 'variation' === $product->get_type() ) : |
5 |
$product_id = $product->get_parent_id(); |
6 |
endif; |
7 |
if ( ! has_term( 'stock-offers', 'product_tag', $product_id ) ) : |
8 |
$valid = false; |
9 |
endif; |
10 |
return $valid; |
11 |
}
|
12 |
add_filter( 'woocommerce_coupon_is_valid_for_product', 'wc_custom_coupon_rules', 10, 4 ); |
With this rule in place, assuming we have available a welcome10 coupon for a 10% discount, a customer with these selections will enjoy a 3.60€ discount.
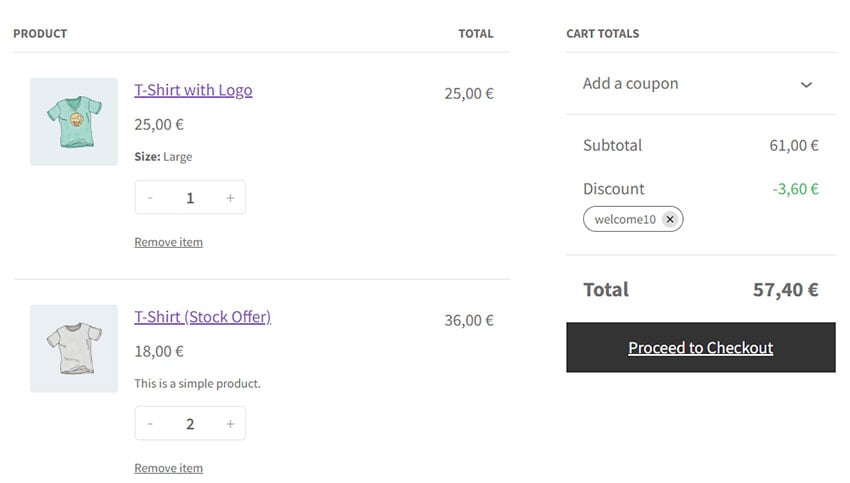
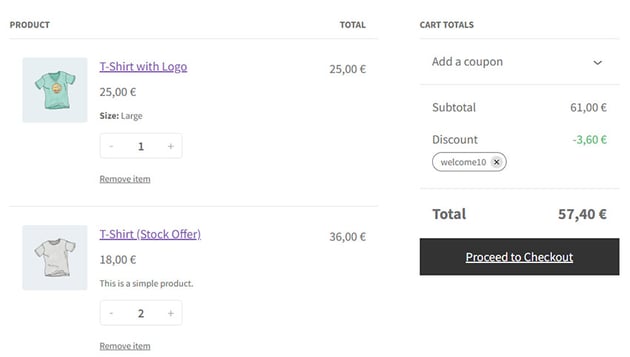
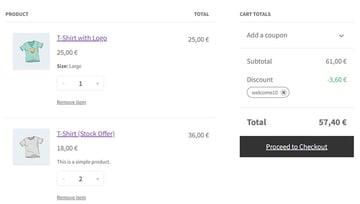
3. Set a discount only for products (simple or variable) without the Stock Offers tag or the Nike brand.
To enable this rule, add the following code in the functions.php
file of your theme:
1 |
<?php
|
2 |
function wc_custom_coupon_rules( $valid, $product, $coupon, $values ) { |
3 |
$product_id = $product->get_ID(); |
4 |
if ( 'variation' === $product->get_type() ) : |
5 |
$product_id = $product->get_parent_id(); |
6 |
endif; |
7 |
if ( has_term( 'stock-offers', 'product_tag', $product_id ) || has_term( 'apple', 'product_brand', $product_id ) ) : |
8 |
$valid = false; |
9 |
endif; |
10 |
return $valid; |
11 |
}
|
12 |
add_filter( 'woocommerce_coupon_is_valid_for_product', 'wc_custom_coupon_rules', 10, 4 ); |
With this rule in place, assuming we have available a welcome10 coupon for a 10% discount, a customer with these selections will enjoy a 2.50€ discount.
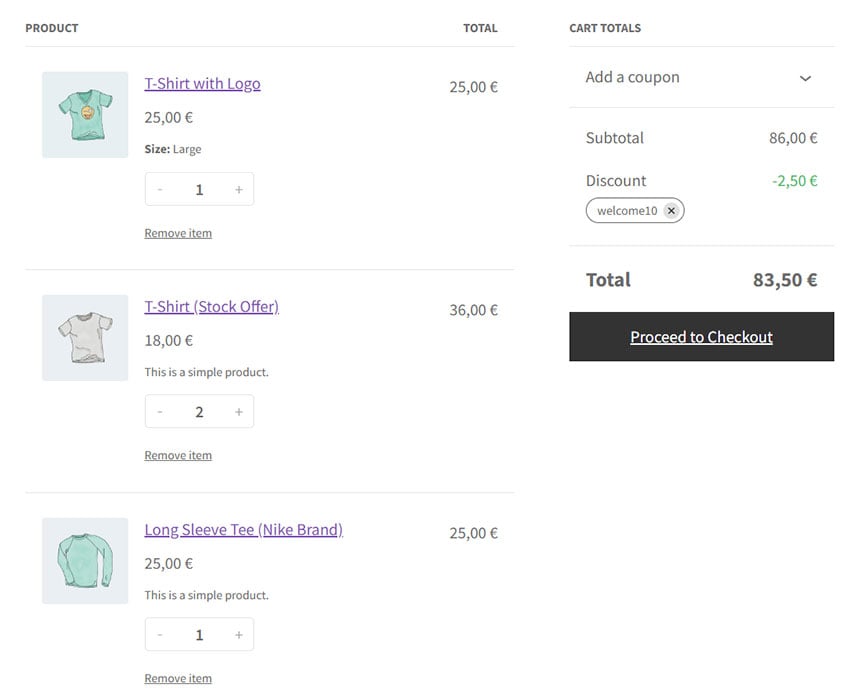
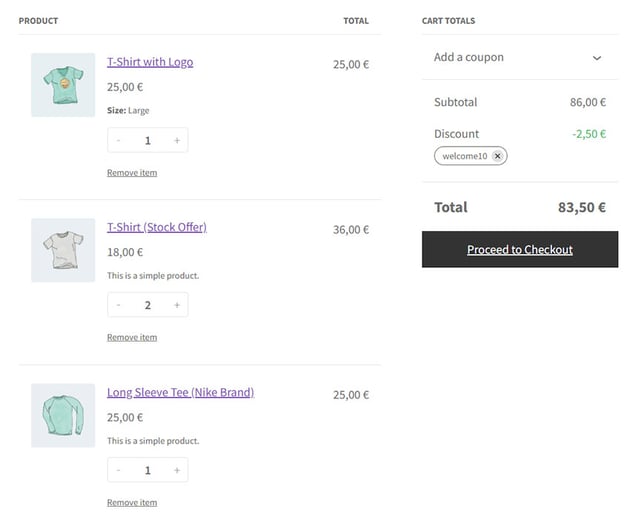
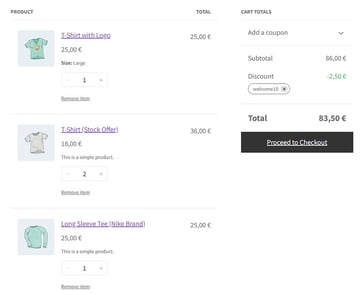
4. Set a discount only for variable products without the Large size attribute.
To enable this rule, add the following code in the functions.php
file of your theme:
1 |
<?php
|
2 |
function wc_custom_coupon_rules( $valid, $product, $coupon, $values ) { |
3 |
if ( 'variation' === $product->get_type() && 'Large' === $product->get_attribute( 'pa_size' ) ) : |
4 |
$valid = false; |
5 |
endif; |
6 |
return $valid; |
7 |
}
|
8 |
add_filter( 'woocommerce_coupon_is_valid_for_product', 'wc_custom_coupon_rules', 10, 4 ); |
With this rule in place, assuming we have available a welcome10 coupon for a 10% discount, a customer with these selections will enjoy a 5€ discount.
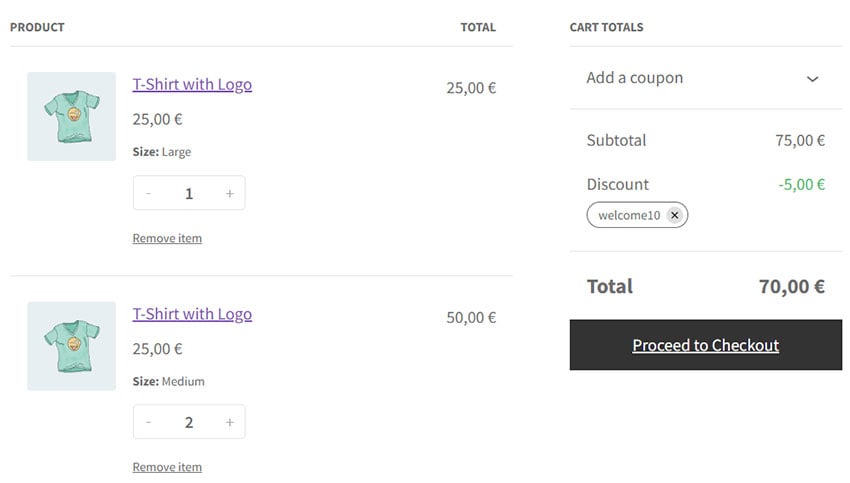
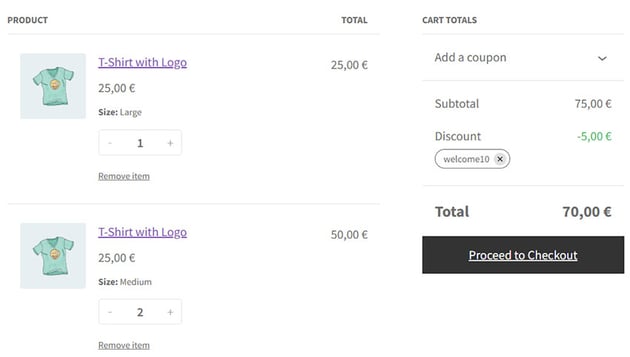
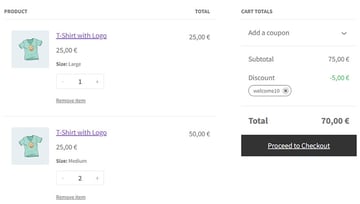
Conclusion
As you can see, with just a small amount of code, we set custom discount rules for different WooCommerce taxonomies.
You can build on the provided code and customize it according to your needs. For example, you can limit some of these conditions only to certain coupons or make the filter options dynamic by adding new fields in the admin. Nothing stops you from combining your own conditions with the ones that WooCommerce provides by default.
As always, thanks a lot for reading!